In today’s dynamic digital landscape, ensuring the quality and dependability of web applications is essential. While Selenium has been a longstanding solution for automating browser tasks, the integration of WebdriverIO (WDIO) with Selenium and JavaScript marks a significant advancement in automation testing. WDIO enhances the testing process by offering a robust interface that improves test creation, execution, and management. This amalgamation capitalizes on the strengths of both tools, leveraging Selenium’s broad browser support and WDIO’s modern, efficient approach to test automation. As automation testing becomes increasingly vital for faster development cycles and superior software releases, WDIO emerges as a versatile framework, particularly potent when paired with JavaScript, making it a preferred choice for contemporary testing teams.
- What is WebdriverIO?
- WebdriverIO Architecture: How Does It Work?
- Why Choose WebdriverIO for Automation Testing?
- Prerequisite Steps to Create a WDIO + JavaScript Project
- ▶️Download & Install Visual Studio Code
- ▶️Install Node.js and npm
- ▶️Create a New Project Directory
- ▶️Install WebdriverIO CLI
- ▶️Run WebdriverIO Configuration Wizard
- ▶️A Configuration steps for setup framework
- ▶️Set Up WebdriverIO Configuration File
- [wdio.conf.js]
- ▶️Create Test Specifications
- [contactUsTests.js]
- Running the Test Script
- Conclusion
What is WebdriverIO?
WebdriverIO is a robust automation testing framework designed for web applications, offering a comprehensive set of features to streamline the testing process. Developed in JavaScript and built on the WebDriver protocol, it provides cross-browser testing capabilities, allowing testers to ensure compatibility across various browser environments such as Chrome, Firefox, Safari, and Edge.With WebdriverIO, testers can interact with web elements effortlessly, performing actions like clicking buttons, typing into text fields, and verifying element visibility. The framework includes built-in assertion libraries and supports external libraries like Chai for assertions and expectations, enabling testers to validate expected outcomes with ease.
WebdriverIO facilitates parallel testing, allowing for simultaneous test execution across multiple browser instances or environments, which significantly reduces test execution time. Moreover, it seamlessly integrates with popular testing frameworks like Mocha, Jasmine, and Cucumber, providing flexibility in test development and structure.
Testers can further extend WebdriverIO’s functionality through custom commands and hooks, enhancing test code reusability and maintainability. Overall, WebdriverIO simplifies the automation testing process, offering a powerful solution for testing web applications with efficiency and reliability.
WebdriverIO Architecture: How Does It Work?
WebdriverIO is developed on NodeJS and utilizes the JSON Wire Protocol for communication. Distributed through npm, WebdriverIO leverages NodeJS, which is open-source and extensively used for application development. It employs a RESTful architecture to facilitate automation testing.
Test scripts are written in JavaScript using the WebdriverIO library. The service request is transmitted via NodeJS as an HTTP command utilizing the JSON Wire Protocol. The services module then forwards the request to the browser.
Upon receiving the command, the browser executes the specified user actions, thereby validating the application’s functionality.
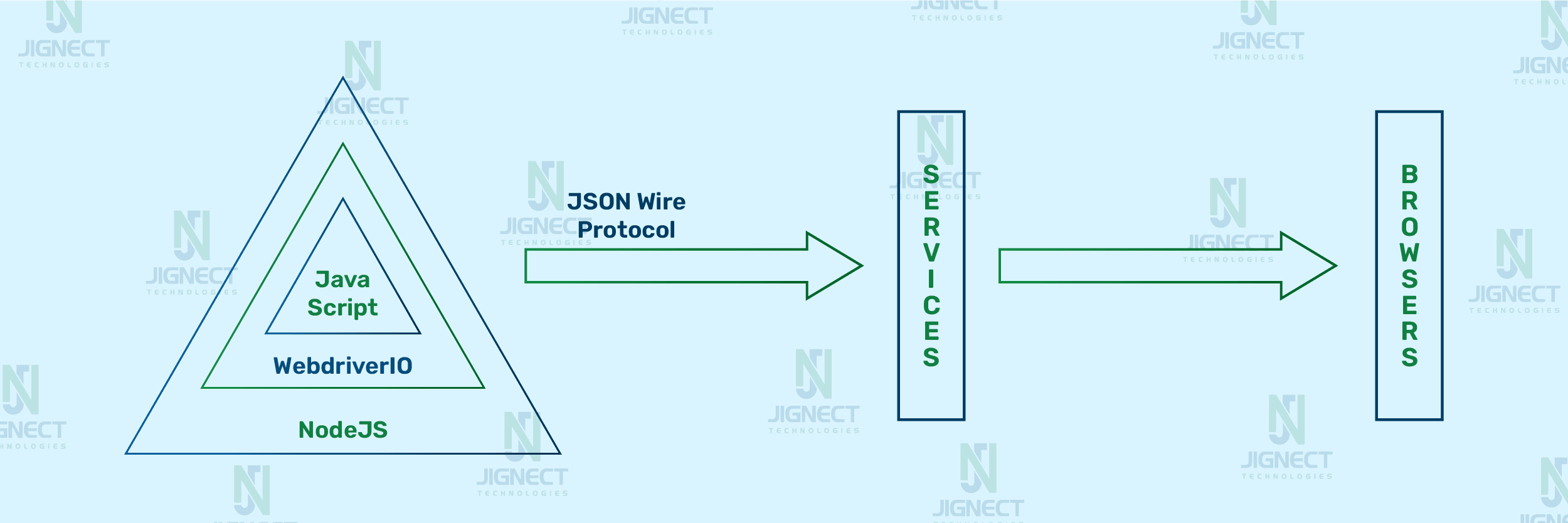
Why Choose WebdriverIO for Automation Testing?
WebdriverIO is a popular choice for automation testing because of its powerful features and easy-to-use interface. Here are some reasons why WebdriverIO is a great option for your automation testing needs:
- JavaScript Integration: WebdriverIO is built using JavaScript, making it a perfect fit for modern web development projects where JavaScript is commonly used. This lets developers use the same language for both development and testing, making the process more efficient.
- Cross-Browser Testing: WebdriverIO supports cross-browser testing, allowing tests to run on different browsers like Chrome, Firefox, Safari, and Edge. This ensures your web application works correctly across various browsers.
- Ease of Use: WebdriverIO has a simple syntax and a well-documented API, making it easy to create and maintain test scripts. Its clear and concise code structure makes it easier for new users to learn.
- Built-In and Extensible Features: WebdriverIO comes with built-in assertion libraries and supports external libraries like Chai for more complex assertions. It also allows adding custom commands and hooks, giving flexibility to meet specific testing needs.
- Parallel Test Execution: WebdriverIO supports parallel test execution, enabling multiple tests to run at the same time. This greatly reduces the total test execution time and increases efficiency.
- Sync/Async Mode: WDIO offers both synchronous and asynchronous modes of test execution. Testers can choose between sync mode, where commands are executed sequentially, and async mode, where commands are executed asynchronously for improved performance.
- Integration with Popular Frameworks: WebdriverIO seamlessly integrates with popular testing frameworks such as Mocha, Jasmine, and Cucumber. This offers flexibility in test development and structure, catering to different testing preferences and styles.
- Strong Community and Support: WebdriverIO has a strong community and active support, providing many resources, tutorials, and plugins. This ensures you can find solutions to common problems and continuously improve your testing practices.
- Comprehensive Reporting: WebdriverIO offers detailed reporting features, including integration with Allure and other reporting tools. This helps in tracking test results, identifying issues, and maintaining comprehensive test documentation.
Prerequisite Steps to Create a WDIO + JavaScript Project
Throughout this blog, the following versions of libraries and applications were utilized in our practical examples.
- Visual Studio Code [Version 1.89]
- Npm [Version 10.5.2]
- Node [20.13.1]
Setting up a WebdriverIO (WDIO) project with JavaScript involves several key steps. Below are the prerequisite steps to get started:
▶️Download & Install Visual Studio Code
- Next, download Visual Studio Code (VS Code) from it’s official site and install it.
▶️Install Node.js and npm
- Ensure that Node.js and npm (Node Package Manager) are installed on your machine. You can download and install Node.js from the official website. Note that npm is bundled with Node.js.
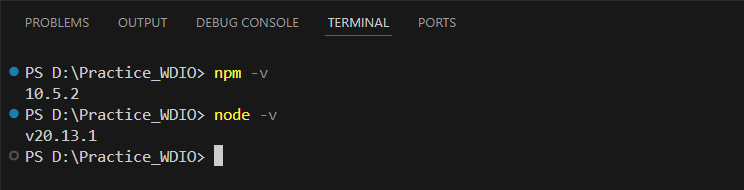
▶️Create a New Project Directory
- Begin by creating a new directory for your project and navigating into it. You can use the following commands for that: “mkdir wdio-js-project” and “cd wdio-js-project”.
- To initialize a WebdriverIO project, hit this command in your project directory terminal: ”npm init wdio@latest .”
- if you want to create a new project in a specific directory, you can use: “npm init wdio@latest ./path/to/new/project”
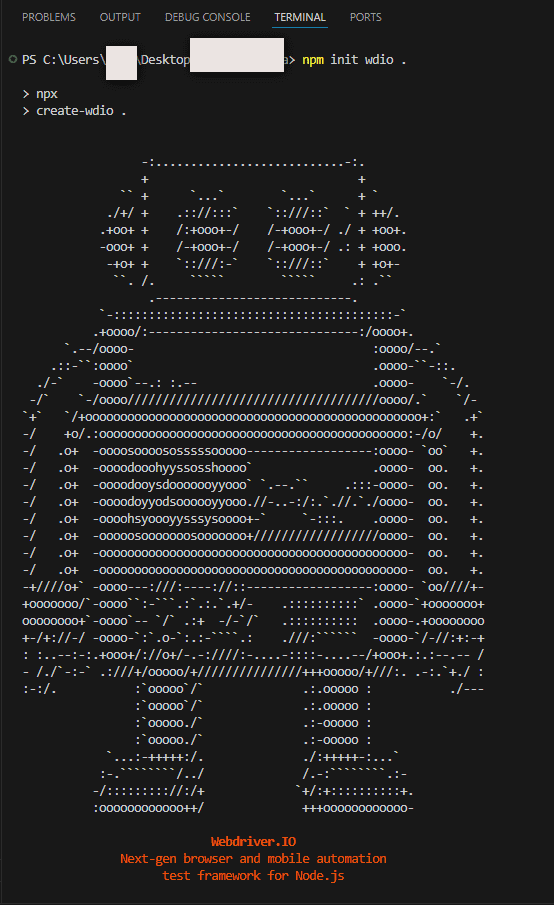
▶️Install WebdriverIO CLI
- Install the WebdriverIO CLI tool globally to facilitate project configuration. Run this cmd command “npm install –save-dev @wdio/cli”.
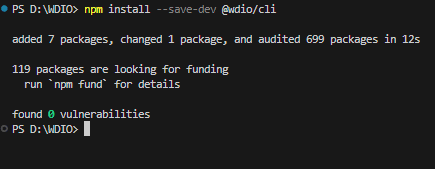
▶️Run WebdriverIO Configuration Wizard
- Execute the WebdriverIO configuration wizard to set up your project. This interactive tool will guide you through selecting your preferred framework, reporter, and other configurations. Hit this “npx wdio config” in the terminal and follow the instructions.
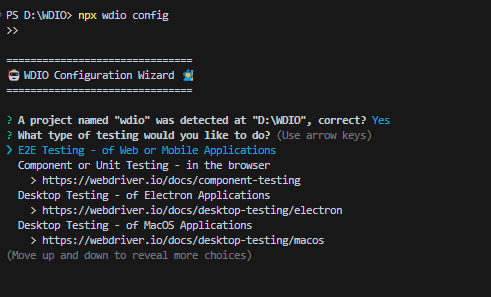
- During configuration, you will be prompted to select various options, such as the test runner (e.g., Mocha, Jasmine, Cucumber), reporter (e.g., Spec, Allure), and services (e.g., Chromedriver, Selenium Standalone, Appium).
▶️A Configuration steps for setup framework
- Depending on your chosen configurations, you need to provide answers as below for the following setup questions based on your requirements.
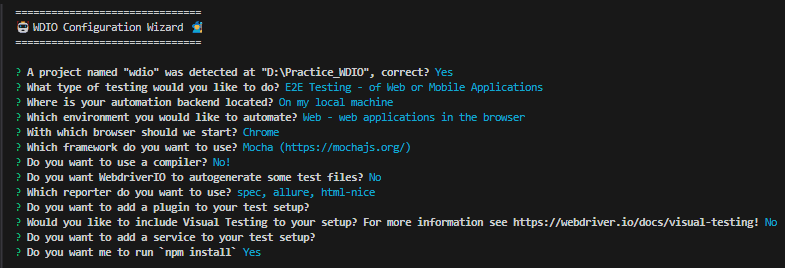
▶️Set Up WebdriverIO Configuration File
Upon finalization, the configuration wizard will produce a “wdio.conf.js” file within your project directory. Take the opportunity to review and adjust this file according to your project requirements. Below is a basic example:
[wdio.conf.js]
export const config = {
runner: 'local',
specs: [
'./test/specs/**/*.js'
],
exclude: [
// 'path/to/excluded/files'
],
maxInstances: 10,
capabilities: [{
browserName: 'chrome'
}],
logLevel: 'info',
bail: 0,
waitforTimeout: 10000,
connectionRetryTimeout: 120000,
connectionRetryCount: 3,
services: ['chromedriver'],
framework: 'mocha',
reporters: ['spec',['allure', {outputDir: 'allure-results'}]],
mochaOpts: {
ui: 'bdd',
timeout: 60000
},
}
▶️Create Test Specifications
Establish a directory for your test specifications (e.g., test/specs) and compose your test scripts in JavaScript. Below is a straightforward example:
[contactUsTests.js]
import { expect, browser, $ } from '@wdio/globals'
describe('Contact Us', () => {
it('verifyThatContactusFormSubmittedSuccessfully', async () => {
await browser.url(`https://jignect.tech/contact-us/`);
await browser.maximizeWindow();
await $("//p//input[@type='submit']").click();
await expect($("//div[contains(@class,'response-output')]")).toBeDisplayed();
})
})
➡️ Let’s analyze each line of the test script and explain its functionality:
- import { expect, browser, $ } from ‘@wdio/globals’: This line imports essential functions and objects from the @wdio/globals package.
- expect is used for assertions.
- browser represents the browser instance to control browser interactions.
- $ is a shorthand for selecting elements using a CSS or XPath selector.
- describe(‘Contact Us’, () => {: This defines a test suite named ‘Contact Us’. The ‘describe function groups the related tests, making it easier to organize and manage them.
- The ‘it’ function defines an individual test case named “verifyThatContactusFormSubmittedSuccessfully” and Used to specify a single test. The ‘async’ keyword allows the use of ‘await’ within the test, enabling asynchronous operations.
- The ‘browser.url’ command navigates the browser to the specified URL (https://jignect.tech/contact-us/). The await keyword ensures that the browser waits for the navigation to complete before proceeding.
- The ‘browser.maximizeWindow’ command maximizes the browser window to ensure that all elements are visible and interactable. Again, await ensures that the test waits for the window to maximize before continuing.
- The await ‘$(“//p//input[@type=’submit’]”).click();’ line selects the submit button and clicks it to submit the form.
- The ‘expect’ and ‘toBeDisplayed()’ assertion verifies that a div element containing the class ‘response-output’ is displayed on the page, indicating that the form submission was successful and closing braces complete the test case and the test suite definitions.
Running the Test Script
Once your test script is prepared, you can execute it by run the following “npx wdio wdio.conf.js” command in the Command Prompt:

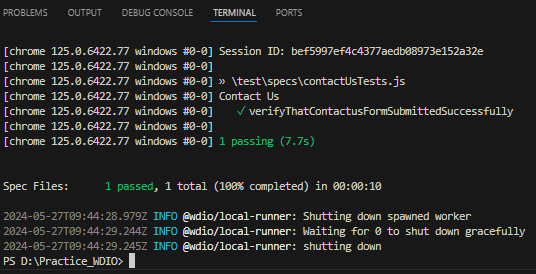
Conclusion
In today’s rapidly evolving digital landscape, ensuring the quality and reliability of web applications is crucial. While Selenium has been a staple for browser automation, integrating WebdriverIO with Selenium and JavaScript brings significant advancements to automation testing. WebdriverIO offers a robust interface for Selenium, enhancing test creation, execution, and management. With its modern approach and extensive browser support, WebdriverIO streamlines the testing process, facilitating faster development cycles and better software releases.
Combining WebdriverIO with JavaScript yields powerful automation solutions. It supports cross-browser testing, simplifies interaction with web elements, and integrates seamlessly with popular testing frameworks. The architecture, built on NodeJS and leveraging the JSON Wire Protocol, ensures efficient communication and execution of tests. Using JavaScript with WebdriverIO provides additional benefits such as a vast ecosystem, asynchronous programming, and strong community support. This enables testers to create scalable and maintainable automation tests, ensuring high-quality web applications.
In conclusion, WebdriverIO, in tandem with JavaScript and Selenium, offers a comprehensive and flexible solution for automation testing, empowering teams to deliver reliable web applications efficiently.
Keep practicing and exploring to master these powerful tools further with Jignect.
Witness how our meticulous approach and cutting-edge solutions elevated quality and performance to new heights. Begin your journey into the world of software testing excellence. To know more refer to Tools & Technologies & QA Services.
If you would like to learn more about the awesome services we provide, be sure to reach out.
Happy Testing 🙂