Playwright is a versatile tool capable of handling both mobile and web applications, providing support for all major browsers. This flexibility allows developers and testers to gain insights into how a web application will look and behave across various screen sizes and under different lighting conditions.
It is important to note that while mobile device emulation in Playwright significantly extends test coverage, it does not replace the need for testing on real devices. Emulation offers a convenient way to perform preliminary checks and ensure that the application adapts well to different device configurations. However, for comprehensive testing, particularly for performance and specific hardware interactions, real device testing remains essential.
By leveraging Playwright’s capabilities, development and QA teams can ensure a more robust and user-friendly experience across a diverse range of devices, ultimately leading to higher quality web applications.
Table of Contents
- Key Capabilities
- Viewport Emulation
- Color Scheme Validation
- Geolocation, Time Zones, and Locales
- Prerequisite Steps
- Initialize the Project
- Create tsconfig.json
- Test case Creation & Result
- How to run a test
- Conclusion
↪️Key Capabilities
Viewport Emulation:
Playwright provides developers with the ability to emulate the viewport sizes of various mobile devices, including both smartphones and tablets. This feature ensures that web applications are responsive and adapt seamlessly to different screen dimensions. By using Playwright’s viewport emulation, developers can catch and address potential issues related to layout and functionality on various devices, ensuring a consistent user experience across all platforms.
Color Scheme Validation:
With the increasing popularity of dark mode and other user-specific color preferences, Playwright’s mobile device emulation capabilities enable testers to validate how a web application’s design performs under different color schemes. This includes checking the consistency and accessibility of the application’s appearance in both light and dark modes. By doing so, developers can ensure that their applications provide a visually pleasing and user-friendly experience regardless of the user’s color scheme preference.
Geolocation, Time Zones, and Locales:
Playwright includes robust features to simulate geolocation, time zones, and locales, facilitating comprehensive testing scenarios. This capability allows developers to test how their web applications function across different geographic regions and time zones, ensuring correct behavior for users worldwide. For instance, by simulating different locales, testers can verify that date and time formats, language settings, and other region-specific features operate as expected. This comprehensive testing helps ensure that the application provides a seamless and accurate experience for users in different parts of the world.
Overall, Playwright’s extensive range of emulation capabilities significantly enhances the testing process, providing developers and testers with powerful tools to ensure their web applications deliver high-quality performance and user experiences across a variety of devices and settings.
Prerequisite Steps
Let’s create a Playwright script in TypeScript to automate the login process on the provided website using mobile device emulation (e.g., iPhone 11).
Initialize the Project:
- npm init -y
- npm install playwright typescript ts-node @types/node
- `npm` : Node Package Manager, a utility for managing JavaScript packages.
- `init` : This initializes a new Node.js project and generates a `package.json` file.
- `-y` : This option automatically responds “yes” to all prompts, accepting the default configurations.
- `npm install` : This command installs the listed packages and includes them as dependencies in your `package.json` file.
Create tsconfig.json:
{
"compilerOptions": {
"target": "ES6",
"module": "commonjs",
"outDir": "./dist",
"rootDir": "./src",
"strict": true,
"esModuleInterop": true
},
"include": ["src/**/*"]
}
Here’s a detailed explanation of each line of the above code:
- compilerOptions:
- Contains various settings that tell the TypeScript compiler how to compile the code.
- target:
- Specifies the version of JavaScript to output.
- Module:
- Defines the module system to use in the output.
- outDir:
- Directory where the compiled files will be placed.
- rootDir:
- Directory containing the source TypeScript files.
- Strict:
- Enables all strict type-checking options.
- esModuleInterop:
- Ensures compatibility between CommonJS and ES modules.
- Include:
- Specifies which files or directories to include in the compilation.
Test case Creation & Result:
Create the TypeScript Script:
Create a src directory and add a file named mobileTest.ts inside it with the following content:
import { chromium, devices } from 'playwright';
const iPhone11 = devices['iPhone 11'];
(async () => {
// Launch the Chromium browser
const browser = await chromium.launch({ headless: false });
// Create a new browser context with the iPhone 11 device settings
const context = await browser.newContext({
...iPhone11,
locale: 'en-US',
geolocation: { latitude: 37.7749, longitude: -122.4194 },
permissions: ['geolocation'],
});
// Create a new page in the context
const page = await context.newPage();
// Navigate to the login page
await page.goto('https://practicetestautomation.com/practice-test-login/');
// Enter username
await page.fill('#username', 'student');
// Enter password
await page.fill('#password', 'Password123');
// Click the login button
await page.click('#submit');
// Check if login was successful by checking for a specific element
const successMessage = await page.textContent('.post-title');
if (successMessage?.includes('Logged In Successfully')) {
console.log('Login successful');
} else {
console.log('Login failed');
}
// Close the browser
await browser.close();
})();
Here’s a detailed explanation of each line of the above code:
- Import Modules
- import { chromium, devices } from ‘playwright’;
- chromium: This imports the Chromium browser from Playwright.devices: This imports predefined device descriptors (e.g., mobile devices) from Playwright.
- Define the Mobile Device
- const iPhone11 = devices[‘iPhone 11’];iPhone11: This line assigns the predefined device descriptor for iPhone 11 to the variable iPhone11. The device descriptor includes information such as the viewport size, user agent, and other settings that emulate the device.
- Asynchronous Function
- (async () => {
- This starts an asynchronous function. The async keyword allows the use of await within the function, enabling asynchronous operations to be performed in a synchronous-looking manner.
- Launch Browser
- const browser = await chromium.launch({ headless: false });
- chromium.launch(): This launches an instance of the Chromium browser.
- { headless: false }: The browser is launched in non-headless mode, meaning the browser window will be visible.
- Create Browser Context with Device Settings
const context = await browser.newContext({
...iPhone11,
locale: 'en-US',
geolocation: { latitude: 37.7749, longitude: -122.4194 },
permissions: ['geolocation'],
});
browser.newContext(): This creates a new browser context with specific settings.
…iPhone11: The spread operator (…) is used to copy all properties from the iPhone11 descriptor into the context settings.
locale: ‘en-US’: Sets the locale (language) to English (United States).
geolocation: { latitude: 37.7749, longitude: -122.4194 }: Sets the geolocation to coordinates corresponding to San Francisco, CA.permissions: [‘geolocation’]: Grants the browser context permission to use geolocation.
- Create New Page
- const page = await context.newPage();
- context.newPage(): This creates a new page (tab) in the browser context.
- Navigate to Login Page
- await page.goto(‘https://practicetestautomation.com/practice-test-login/‘);
- page.goto(): This navigates to the specified URL, in this case, the login page of the provided website.
- Enter Username
- await page.fill(‘#username’, ‘student’);
- page.fill(): This fills in a form field identified by the CSS selector #username with the text ‘student’.
- Enter Password
- await page.fill(‘#password’, ‘Password123’);
- page.fill(): This fills in a form field identified by the CSS selector #password with the text ‘Password123’.
- Click the Login Button
- await page.click(‘#submit’);
- page.click(): This clicks on a button identified by the CSS selector #submit.
- Check Login Success
const successMessage = await page.textContent('.post-title');
if (successMessage?.includes('Logged In Successfully')) {
console.log('Login successful');
} else {
console.log('Login failed');
}
page.textContent(): This retrieves the text content of an element identified by the CSS selector .post-title.
successMessage?.includes(‘Logged In Successfully’): This checks if the retrieved text content includes the phrase ‘Logged In Successfully’.
console.log(‘Login successful’): Logs ‘Login successful’ to the console if the phrase is found.console.log(‘Login failed’): Logs ‘Login failed’ to the console if the phrase is not found.
- Close Browser
- await browser.close();
- })();
- browser.close(): This closes the browser.})();: This ends the asynchronous function and immediately invokes it.
How to run a test
- Open the terminal and hit the command: npx ts-node src/mobileTest.ts

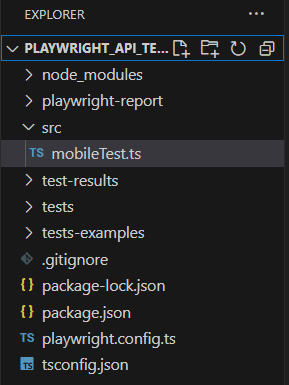
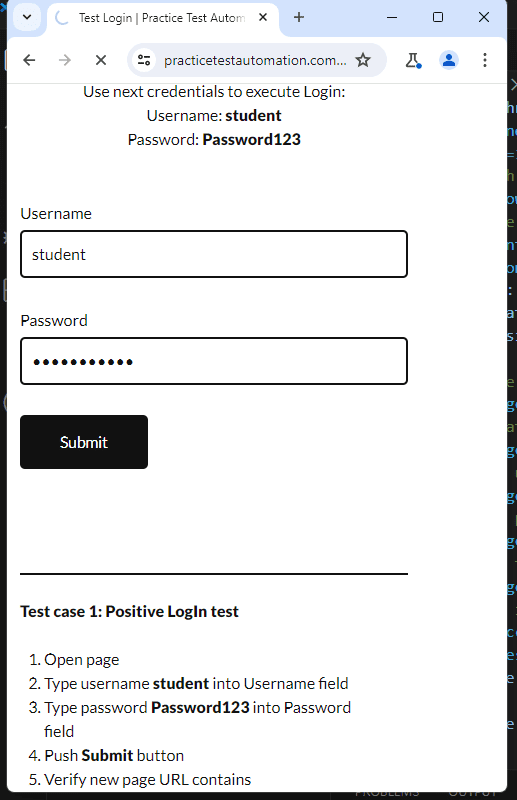
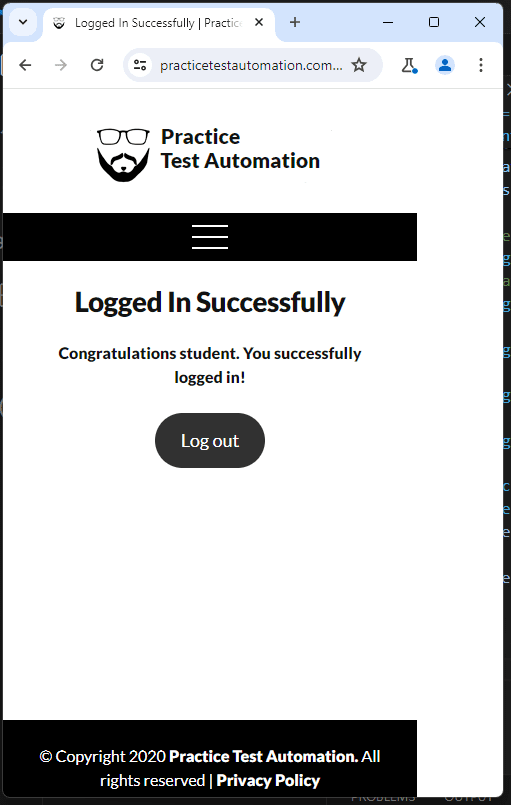

Conclusion
In this project, we built a robust testing framework using Playwright and TypeScript to automate a website’s login functionality. By emulating an iPhone 11, we ensured the site performs well on mobile devices. The TypeScript configuration (`tsconfig.json`) optimized code compilation, and the script (`loginTest.ts`) automated the entire login process, from entering credentials to verifying success.
This method streamlines testing and enhances website quality by uncovering issues in a mobile environment. Leveraging Playwright and TypeScript, we developed a reliable, scalable testing solution. However, it’s important to note that mobile device emulation does not replace testing on real devices, and the initial setup can be complex.
Keep practicing and exploring to master these powerful tools further with Jignect.
Witness how our meticulous approach and cutting-edge solutions elevated quality and performance to new heights. Begin your journey into the world of software testing excellence. To know more refer to Tools & Technologies & QA Services.
If you would like to learn more about the awesome services we provide, be sure to reach out.
Happy testing! 🙂