Incorporating Automated Testing with Python Selenium
In today’s fast-paced software development scenario, Automation testing is crucial for maintaining application quality and reliability. Python shines as a top choice among various automation tools, notably in combination with Selenium for web automation and the unittest framework for test case administration. This blog will cover Python basics, its suitability for Selenium automation, the significance of the unittest framework, and the setup process for writing and running Python scripts in PyCharm for automation testing. Additionally, we will explore how to use Selenium unittest for seamless integration and Python unittest assertions for validating test outcomes.
What is Python?
Python is a high-level, interpreted programming language known for its focus on code readability through significant indentation. It is dynamically typed and garbage-collected, supporting various programming paradigms such as structured, object-oriented, and functional programming. Python provides a wide range of modules and packages for various tasks without needing additional installation. Python is applicable in various areas such as web development, data analysis, automation, and artificial intelligence.
Why Selenium?
Selenium is an open-source tool widely used for automating web browsers. It provides a suite of tools for web application testing across different browsers and platforms. Selenium WebDriver, in particular, allows users to control web browsers programmatically, enabling the creation of powerful automated tests.For example, combining Python Selenium can significantly streamline your testing processes.
Here are some reasons why Selenium is a preferred choice for test automation:
- Cross-browser Compatibility : Selenium WebDriver supports multiple browsers, including Chrome, Firefox, Safari, and Edge, ensuring comprehensive test coverage across different environments.
- Language Support : Selenium WebDriver provides compatibility with several programming languages, including Java, Python, C#, and JavaScript. This flexibility allows testers to work with their preferred programming language.
- Rich Ecosystem : Selenium has a vast ecosystem with a thriving community, providing access to numerous resources, plugins, and integrations to enhance the testing process.
- Element Interaction: Selenium provides mechanisms to locate elements on a web page using various strategies such as ID, name, XPath, CSS selectors, etc. This enables testers to interact with specific elements accurately.
- Handling Alerts and Pop-ups: Selenium can handle alerts, pop-ups, and dialog boxes that appear during the testing process, allowing for more comprehensive automation scenarios.
- Dynamic Web Element Handling: Selenium supports handling dynamic elements on web pages, such as those generated by JavaScript frameworks like AngularJS, React, or Vue.js
- Testing Framework Integration: Selenium can be integrated with popular testing frameworks such as JUnit, TestNG, NUnit, and Pytest, providing additional functionalities like reporting, parallel execution, and data parameterization.
- Integration with Continuous Integration (CI) Tools: Selenium can be seamlessly integrated into CI/CD pipelines using tools like Jenkins, Travis CI, or CircleCI, allowing for automated testing as part of the software development lifecycle.
For further insights into Selenium, you can check out its official documentation.
Why do we use Selenium with Python?
- Selenium is a tool that helps automate interactions with web browsers. It works well across different platforms and browsers, and can be easily used with Python.
- Python has other useful libraries like Requests and Pandas that can be combined with Selenium to make web automation tasks more flexible and effective.
- By using Selenium with Python, you can easily integrate it with Python frameworks to handle complex tasks such as grouping test cases, setting up environments, cleaning up after tests, and making assertions.
What is unittest framework?
- The Python unit testing framework, known as PyUnit, is the Python version of JUnit, supporting test automation and sharing. It offers features like fixtures, test cases, test suites, and a test runner for automated testing, allowing execution from modules, classes, or individual test methods.
Prerequisite for selenium + python automation scripts:
In this blog, during the practical, we’ve used below versions for respective languages, framework, package & applications:
- Python version: 3.12.0
- Selenium version: 4.19.0
- Pip version: 24.0
- Pycharm version: 2023.3.5
Download and Install Python:
- To get started, Download Python, install it with guidance of installation steps.
- Once installation is done, you can verify by entering below command in the command prompt which displays the installed python version details.
- python —-version
- You can verify that pip is installed along with Python by entering the below command in the command prompt.
- pip —-version
Install Selenium:
- If you intend to execute the code using the system command prompt, it is crucial to install the Selenium framework by executing the below command in the command prompt.
- pip install selenium
- After completing the Selenium installation. You can confirm the installation by utilizing the below command.
- pip list
Download and Install Pycharm Editor:
- To download Pycharm, visit the official website and click on the download button of the community edition.
- Once download is done, double click on .exe file and proceed with installation.
- For guidance on installation you can refer to the Install PyCharm site.
Test Case Creation
1. PyCharm Project Setup:
- Launch ‘PyCharm’ and click on ‘New Project’.
- Note: Select ‘Do not import settings’ if installed for the first time.
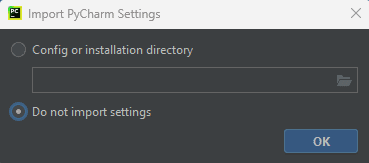
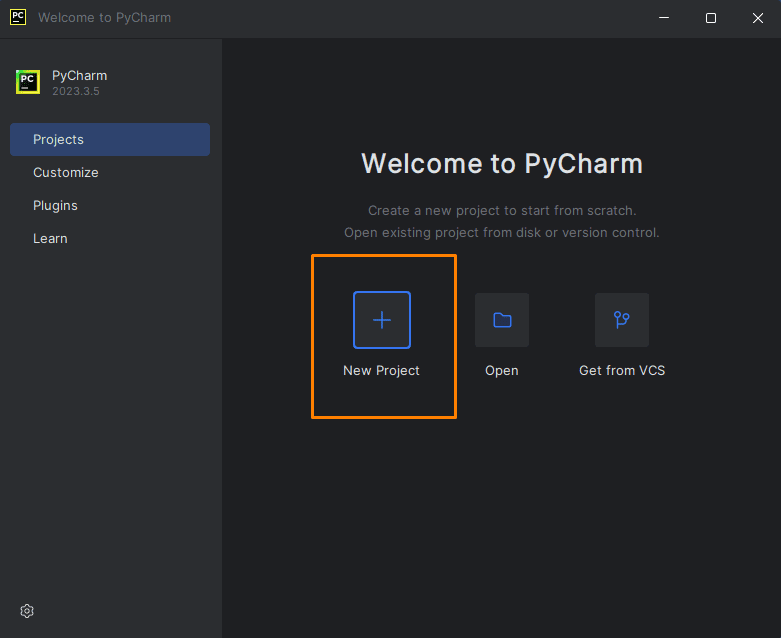
- Enter the project name, choose the location of the project, Interpreter type as Project venv and click on the ‘Create’ button.
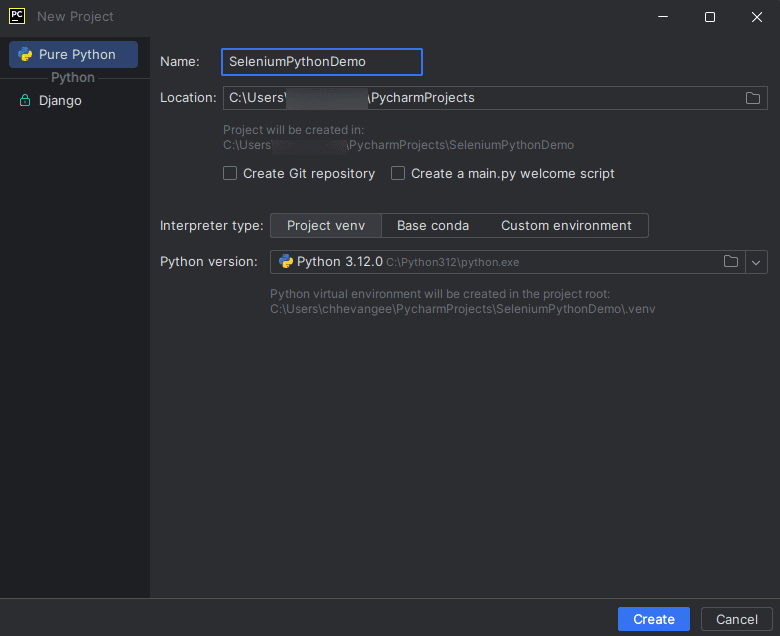
- Ensure that your created project is displayed like the image below.
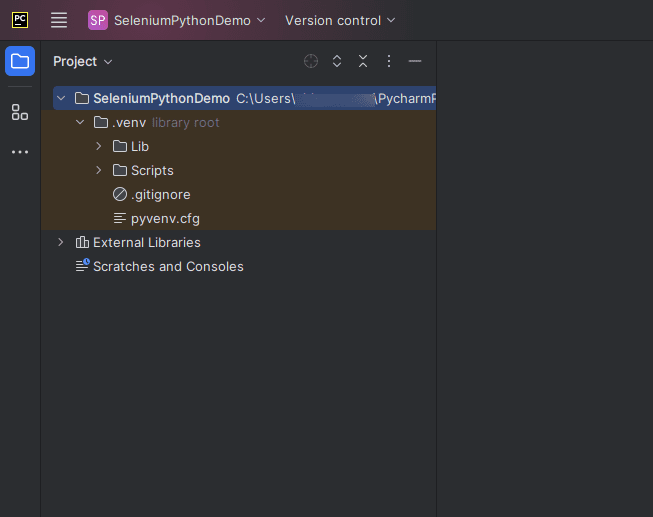
2. Install selenium package:
You can install the Selenium package in your project using either of the following two methods:
- Package setup using icon:
- Click the ‘Python Package’ icon at the bottom left of the IDE, search for ‘Selenium’ package, select it, click ‘Install’, and choose the desired version.
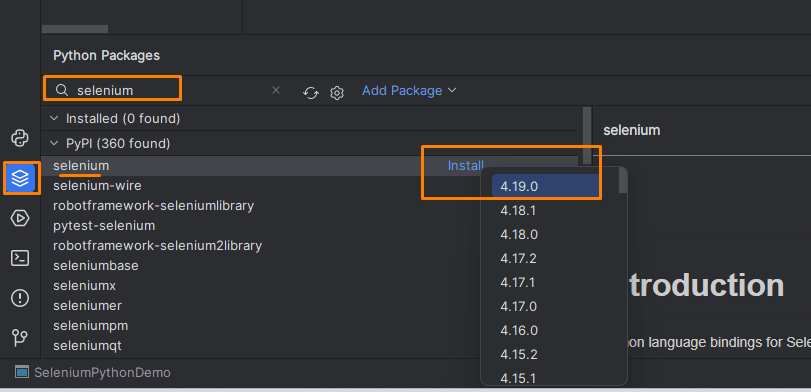
OR
- Package setup using settings:
- Open ‘Settings’ from the ‘File’ menu or ‘Ctrl+Alt+s’ shortcut key.
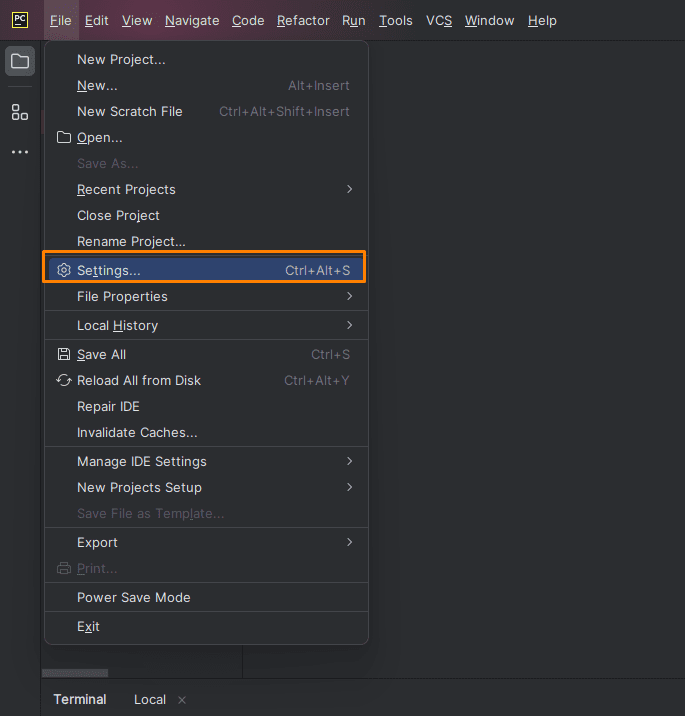
- Click on ‘Project: projectName’, select ‘Python Interpreter’ and click on ‘+’ icon of ‘Python Interpreter’ section.
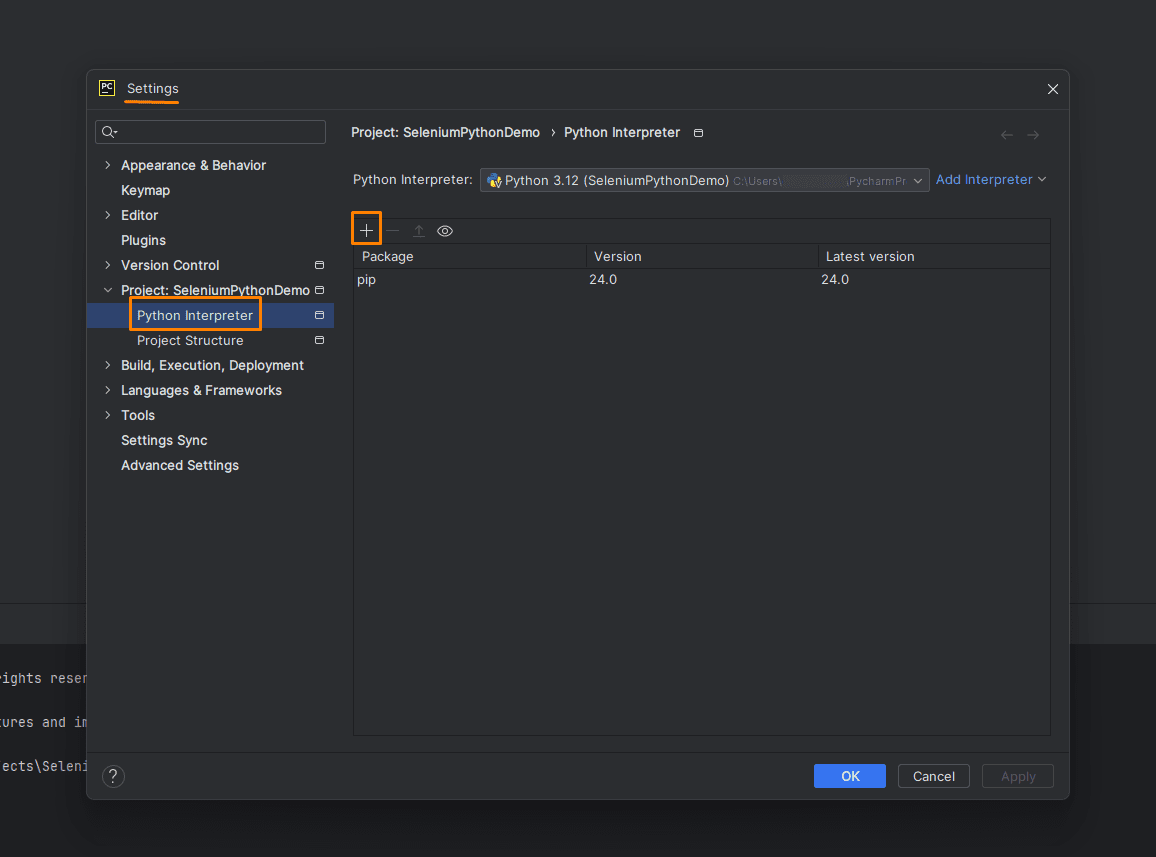
- Search package ‘Selenium’, select and click on the ‘Install Package’ button.
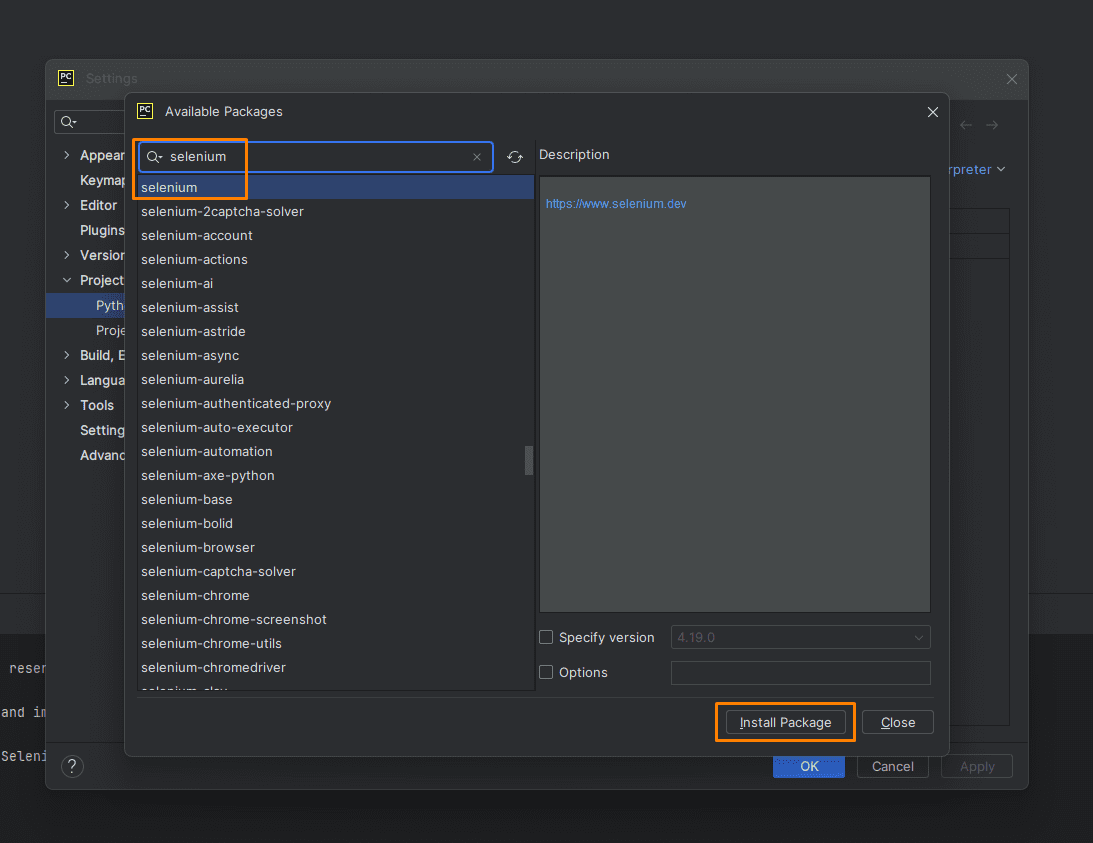
3. Create python file and write test case:
- Create a new Python file with the name ‘LinearDemo’ by selecting ‘Python File’ from the ‘File > New’ menu or by right-clicking on the project name and selecting ‘New’.
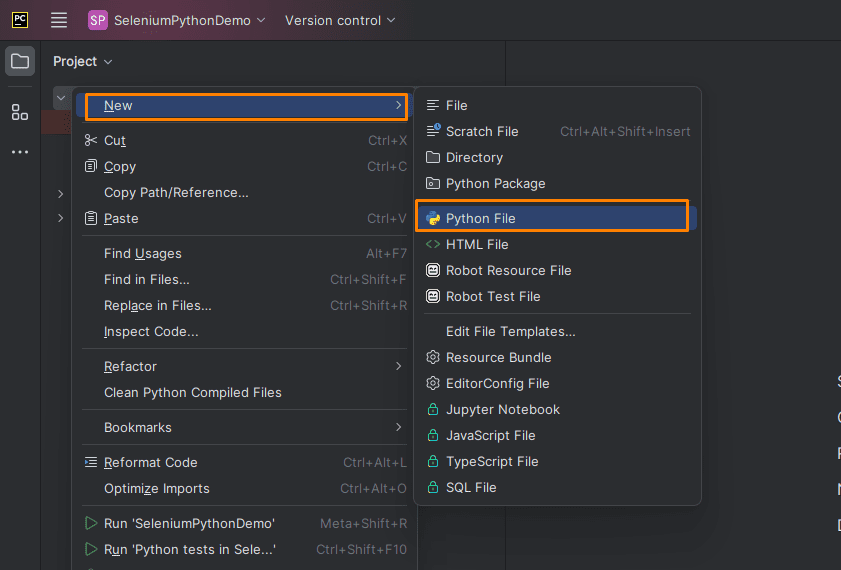
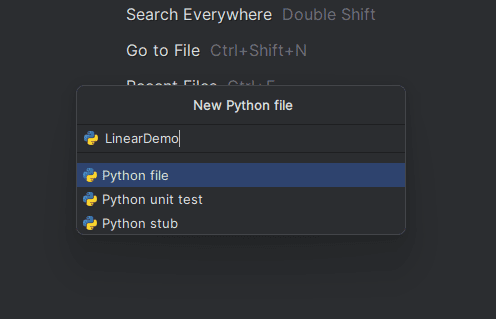
- Once a python file is created, start writing basic test code with the help of selenium and unittest framework as described below.
# Import the necessary packages
import unittest
import random
import string
from selenium import webdriver
from selenium.webdriver.common.by import By
# Class Create
class LinearDemo(unittest.TestCase):
def setUp(self):
"""Setup method to initiate the Chrome browser."""
self.driver = webdriver.Chrome()
self.driver.maximize_window()
self.driver.implicitly_wait(2)
def test_linear_flow_testing(self):
# Navigate to desired web page and verify web page is displayed
self.driver.get("https://jignect.tech/")
self.assertTrue("Software Testing Company" in self.driver.title)
# Click on the contact us button
contact_us_button = self.driver.find_element(By.XPATH, "//ul[@id='menu-main-menu']//a[text()='Contact Us']")
contact_us_button.click()
# Verify desired page is displayed
contact_us_header = self.driver.find_element(By.CSS_SELECTOR, "div[class='contact-title'] h2").text
self.assertEqual(contact_us_header, "Let's talk", "Contact Us page does not displayed")
# Generate random string
full_name = "".join(random.choice(string.ascii_letters) for _ in range(8))
# Enter value in full name and company name, select checkbox and click on submit button
self.driver.find_element(By.CSS_SELECTOR, "input[placeholder='Full name']").send_keys(full_name)
self.driver.find_element(By.CSS_SELECTOR, "input[placeholder='Company']").send_keys("TechTonic")
self.driver.find_element(By.CSS_SELECTOR, "span[id='checkboxOne']").click()
self.driver.find_element(By.CSS_SELECTOR, "input[value='Submit']").click()
# Verify the validation message for mandatory fields
expected_validation_message = 'The field is required.'
textarea_actual_validation_message = self.driver.find_element(By.CSS_SELECTOR,
"textarea[name*='textarea'] + span").text
email_actual_validation_message = self.driver.find_element(By.CSS_SELECTOR, "input[name*='email'] + span").text
phone_number_actual_validation_message = self.driver.find_element(By.CSS_SELECTOR,
"input[placeholder='Phone number'] + span").text
self.assertEqual(textarea_actual_validation_message, expected_validation_message,
"Textarea validation message does not match")
self.assertEqual(email_actual_validation_message, expected_validation_message,
"Email validation message does not match")
self.assertEqual(phone_number_actual_validation_message, expected_validation_message,
"Phone number validation message does not match")
def tearDown(self):
"""Tear down method to close the browser."""
self.driver.quit()
# It is mandatory when you want to run code using command prompt
if __name__ == '__main__':
unittest.main()
This test script utilizes unittest.TestCase class from unittest package to perform automation testing on the JigNect website. Here’s a brief explanation of unittest.TestCase class.
- The unittest.TestCase class is used to create test cases by subclassing it. It provides a set of testing methods and assertions to verify the behavior and output of functions and methods within a Python program.
- Define testing methods within the test case class, with each method name starting with “test_” to be recognized as a test case.
- unittest.TestCase offers assertions such as assertEqual, assertTrue, assertRaises to check website output against the expected result.
- The functions ‘setup’ and ‘teardown’ serve as beforeMethod and afterMethod annotations, running before and after each test case respectively.
- Unittest.main() is essential to include at the end of the class for running code via the command line.
If you’re interested in learning more about unittest, visit its official website for additional information.
Overall, this test script ensures that the website navigation and page redirection and contact us form work as expected, using assertions to validate the expected outcomes at each step.
Run the created Test Case and check result:
- Once the test script is written, proceed to execute the test and carefully analyze the results.
- To run the test case, go to the test class or main method or test method click or right-click on it, and choose the “Run” option. The results will be displayed in PyCharm’s Run tool window.
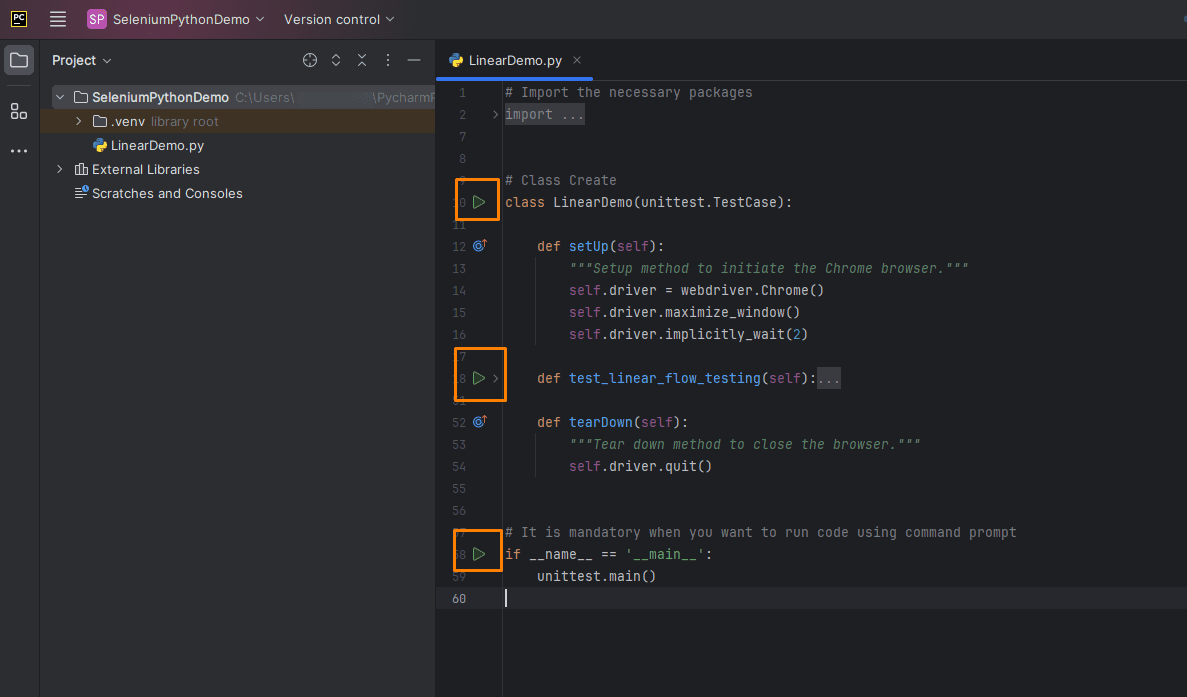
- Test Run Results
- The results shown in the PyCharm Run tool window provide important information about the test run.
- As you can see, the test we created has passed and is functioning correctly as anticipated.
- By reviewing these results, we can understand which tests passed, failed, or were skipped.
- This helps us improve our testing process and make our scripts better and more precise.
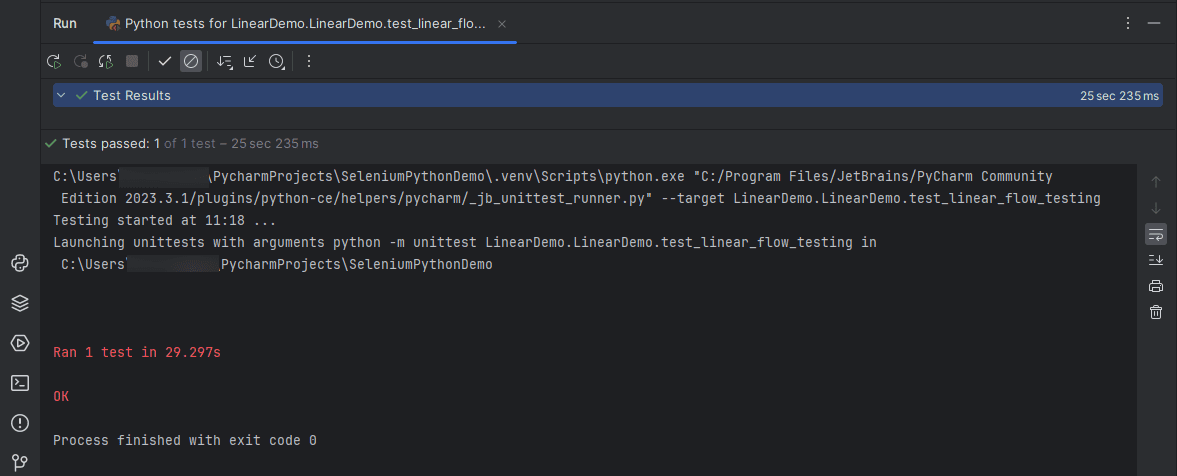
Conclusion:
Python, with its built-in `unittest` framework, offers a straightforward and comprehensive approach to test automation. The framework’s simple syntax, support for assertion methods, and automated test discovery make it easy to write and execute tests. Additionally, the ability to organize test cases into suites and integrate with test runners enhances reusability and streamlines the testing process, making Python and `unittest` an efficient choice for automation testing. However, there are some shortcomings such as the deprecation of returning values from test methods other than the default None value in the version 3.11, and challenges in managing larger test suites as certain development patterns become less efficient.
we’ve gained knowledge about why we use python with selenium and unittest framework with a basic example in our blog. In future blog posts, we will write about python and unittest framework topics such as page object model, data factories, fixtures, reporting, test suits and many more. Stay tuned for more automation-related content in our upcoming blog posts!
Keep practicing and exploring to master these powerful tools further with Jignect.
Witness how our meticulous approach and cutting-edge solutions elevated quality and performance to new heights. Begin your journey into the world of software testing excellence. To know more refer to Tools & Technologies & QA Services.
If you would like to learn more about the awesome services we provide, be sure to reach out.
Happy Testing 🙂