LINQ in C# is used to work with data access from sources such as objects, data sets, SQL Server, and XML. LINQ stands for Language Integrated Query.
To enhance your data manipulation capabilities, you can use LINQ (Language Integrated Query) in C#.
In general, these tools help you to perform various data operations, such as filtering, conditional data retrieval, and data type conversion.
LINQ can be used with various data sources, including: Arrays, Lists, JSON, XML, docs, collections, ADO.Net DataSet, Web Service, MS SQL Server, and different database servers.
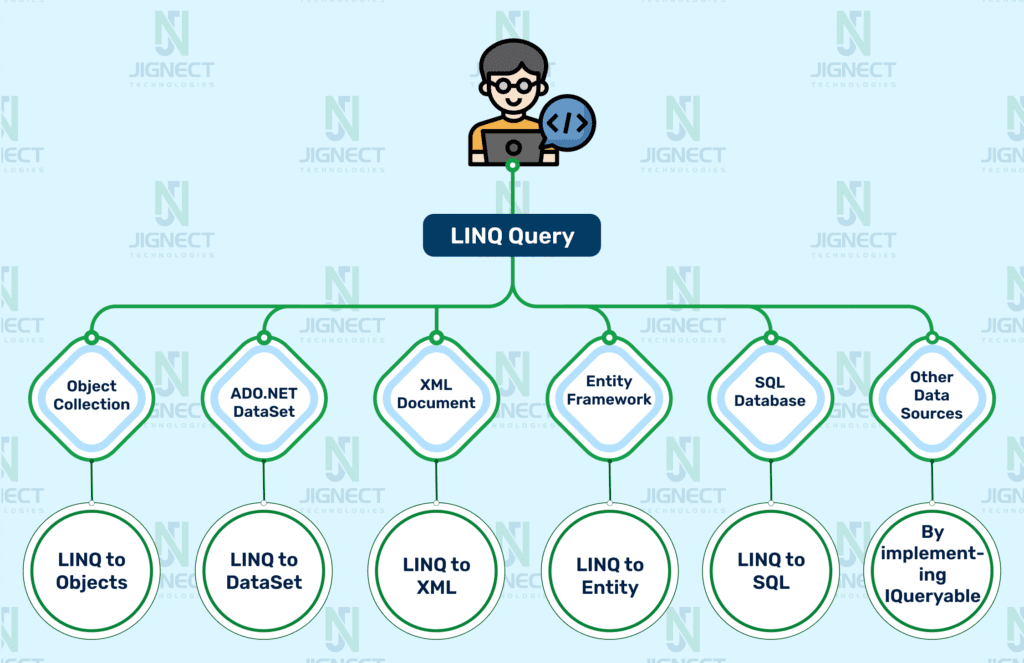
Let’s understand why LINQ was created and how we benefit from using it. These points will clarify its purpose.
- The main purpose behind creating LINQ is, before C# 3.0 we use for loop, foreach loop, or delegates traverse a collection to find a specific object, but the disadvantage of using these methods for finding an object is you need to write a large sum of code to find an object which is more time-consuming and makes your program less readable. So to overcome these problems LINQ introduced. Which performs the same operation in a few numbers of lines and makes your code more readable and also you can use the same code in other programs.
- It also provides full type checking at compile time, it helps us to detect the error at the runtime, so that we can easily remove them.
- LINQ is simple, well-ordered, and high-level language than SQL.
- You can also use LINQ with C# array and collections. It gives you a new direction to solve the old problems in an effective manner.
- With the help of LINQ you can easily work with any type of data source like XML, SQL, Entities, objects, etc. A single query can work with any type of database no need to learn different types of languages.
- LINQ supports query expression, Implicitly typed variables, Object and collection initializers, Anonymous types, Extension methods, and Lambda expressions.
👉 Query Expressions
- Query expressions are at the heart of LINQ. They allow you to express your queries in a way that closely resembles natural language. A query expression typically consists of the following parts:
- From: Specifies the data source.
- Where: Filters data based on a condition.
- Select: Projects data into a new form.
- Group By: Groups data based on a common attribute.
- Order By: Sorts data in a specified order.
using System;
using System.Collections.Generic;
using System.Linq;
namespace ConsoleAppPrograms
{
internal class Person
{
public string Name { get; set; }
public int Age { get; set; }
public string City { get; set; }
}
internal class Program
{
private static void Main()
{
List<Person> people = new List<Person>
{
new Person { Name = "Nehal", Age = 25, City = "Ahmedabad" },
new Person { Name = "Aarti", Age = 27, City = "Rajkot" },
new Person { Name = "Dhwani", Age = 22, City = "Bharuch" },
new Person { Name = "Rajan", Age = 35, City = "Mehsana" },
new Person { Name = "Chandan", Age = 28, City = "Surat" },
new Person { Name = "Ravi", Age = 40, City = "Baroda" }
};
// LINQ query using Query Expression
var queryResult = from person in people
where person.Age > 25
orderby person.Name descending
select new
{
person.Name,
person.City
};
// Query execution
foreach (var result in queryResult)
{
Console.WriteLine($"Name: {result.Name}, City: {result.City}");
}
}
}
}
👉 Lambda Expressions
- Lambda expressions are used in LINQ to define inline, anonymous functions. They are often employed with Where, Select, and other LINQ operators to express filtering and projection conditions succinctly.
using System;
using System.Collections.Generic;
using System.Linq;
namespace ConsoleAppPrograms
{
internal class Person
{
public string Name { get; set; }
public int Age { get; set; }
public string City { get; set; }
}
internal class Program
{
private static void Main()
{
List<Person> people = new List<Person>
{
new Person { Name = "Nehal", Age = 25, City = "Ahmedabad" },
new Person { Name = "Aarti", Age = 27, City = "Rajkot" },
new Person { Name = "Dhwani", Age = 22, City = "Bharuch" },
new Person { Name = "Rajan", Age = 35, City = "Mehsana" },
new Person { Name = "Chandan", Age = 28, City = "Surat" },
new Person { Name = "Ravi", Age = 40, City = "Baroda" }
};
// LINQ query using Lambda Expression
var queryResult = people
.Where(person => person.Age > 25)
.OrderByDescending(person => person.Name)
.Select(person => new
{
person.Name,
person.City
});
// Query execution
foreach (var result in queryResult)
{
Console.WriteLine($"Name: {result.Name}, City: {result.City}");
}
}
}
}
Certainly! This C# program uses LINQ to filter, order, and project data from a list of Person objects. It selects names and cities of people older than 25, orders them by name in descending order, and prints the results.
Witness how our meticulous approach and cutting-edge solutions elevated quality and performance to new heights. Begin your journey into the world of software testing excellence. To know more refer to Tools & Technologies & QA Services.
If you would like to learn more about the awesome services we provide, be sure to reach out.
Happy Testing 🙂