Introduction:
Playwright is a highly powerful and open-source test automation framework designed by Microsoft for testing web applications. It offers robust capabilities for performing end-to-end testing with exceptional efficiency and accuracy. Playwright enables developers to automate tasks across multiple browsers, including Chrome, Firefox, Safari, and Microsoft Edge, ensuring seamless automation and reliable test results. The framework handles various testing scenarios, from simple user interactions to complex workflows, providing a consistent testing experience across different browser environments.
Playwright’s user-friendly interface and extensive documentation make it accessible to both novice and experienced testers. It supports a wide range of testing strategies, helping developers streamline their testing processes and improve the overall quality of their web applications. By leveraging its powerful features, developers can achieve higher test coverage and reduce the time and effort required for manual testing. Additionally, Playwright’s integration with popular CI/CD tools and its support for parallel test execution further enhance its efficiency, making it a valuable asset for any development team focused on delivering high-quality software.
What is Playwright and why is it used in API testing?
Playwright is an open-source end-to-end test automation framework derived from Puppeteer, based on Node.js and managed by Microsoft. It utilizes a single WebSocket to communicate with all drivers, making Playwright one of the fastest and easiest automation frameworks in the software testing world. The framework is remarkably simple to set up, requiring just a single line of code to establish the automation environment. Playwright supports multiple languages, including JavaScript, Java, Python, and .NET (C#). Additionally, it is compatible with a wide range of test frameworks such as Mocha, Jest, Jasmine, and Cucumber.
Playwright is used for single-page applications (SPAs) and progressive web apps (PWAs) that perform data retrieval and other operations in the background. For API testing, Playwright uses the `APIRequestContext` class to send HTTP requests over the network. The `APIRequest` class is utilized to create instances of `APIRequestContext`. These API request instances are created by Playwright Request.
For an in-depth understanding of the main features and capabilities of Playwright with Typescript, you can refer to our previous blog on Playwright.
Prerequisite steps
To configure Playwright with TypeScript, follow these steps:
▶️Create a Project Folder:
Create a sample folder for your project and open it in Visual Studio Code.
▶️Open Terminal:
Open the terminal in Visual Studio Code. To configure Playwright with TypeScript, you need to install the Playwright library and the TypeScript compiler. This can be done using the following command:
npm init playwright@latest
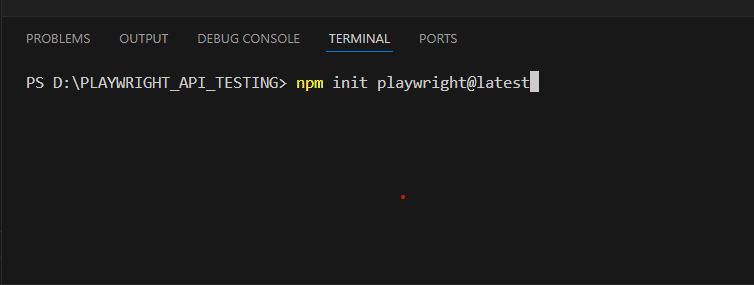
▶️Install Node.js and NPM:
If Node.js and NPM (Node Package Manager) are not already installed on your system, you need to download and install them from the official Node.js website. You can easily download it from this link: Node.js Download.
▶️Configuration Prompts:
After running the above command, the installation process will prompt you to configure certain settings:
▶️Select TypeScript or JavaScript:
Choose TypeScript (the default option is TypeScript).
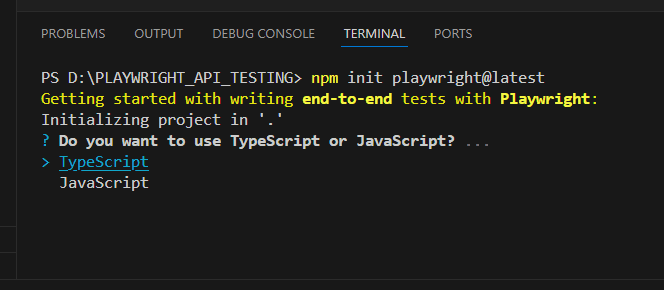
▶️Name Your Test Folder:
You will be asked to name your test folder. The default folder is usually tests or e2e if you already have a test folder in your project.
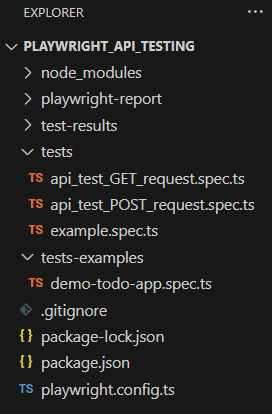
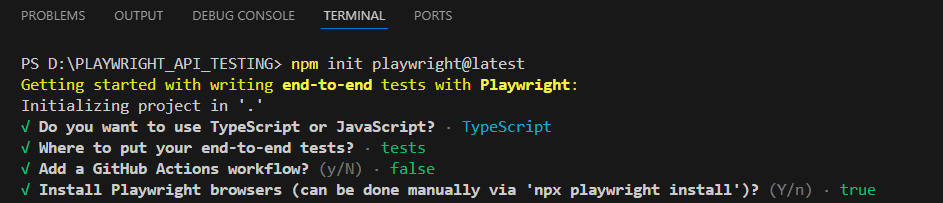
▶️GitHub Integration:
You will also have the option to add GitHub integration, which is optional.
- Following these steps will set up Playwright with TypeScript in your project, enabling you to start writing and running your tests efficiently.
- After setting up Playwright with TypeScript, you can perform a GET request to an API and retrieve the response. In this example, we will use the reqres.in API, which provides a set of endpoints for testing purposes.
Test case Creation & Result:
↪️To create a test file and perform a GET request using Playwright, follow these detailed steps:
Create the Test File:
- First, ensure you have a folder designated for your test files. If you haven’t already, create a folder named tests (or another name of your choice) in your project directory.
- Within this folder, create a new file named api.test.ts.
import {test, expect} from '@playwright/test';
import { request } from 'http';
test('GET API request',async({request}) =>{
const response = await request.get('https://reqres.in/api/users/2')
expect(response.status()).toBe(200)
console.log(await response.json());
})
Explanation of above code:
- import {test, expect} from ‘@playwright/test’;
- The above import line is the test and expect functions from the Playwright test library. test is used to define a test case, and expect is used for assertions within the test.
- import { request } from ‘http’;
- The above import line is request module from the Node.js http package, which is used to make HTTP requests. However, this import is actually not necessary in this context because the request object is provided by Playwright within the test function. This line can be omitted.test(‘GET API request’, async ({ request }) => {
- This line defines a new test case named ‘GET API request’. The async keyword indicates that the function will contain asynchronous code. The { request } parameter is destructured from the Playwright context, providing an instance of the Playwright request object for making HTTP requests.
- const response = await request.get(‘https://reqres.in/api/users/2‘)
- This line performs an asynchronous GET request to the specified URL (https://reqres.in/api/users/2) using the Playwright request object. The await keyword ensures that the code waits for the request to complete before proceeding. The response from the API call is stored in the response variable.
- expect(response.status()).toBe(200)
- This line uses the expect function to assert that the status code of the response is 200, which indicates a successful request. The status() method of the response object returns the HTTP status code of the response.
Run the Test Results:
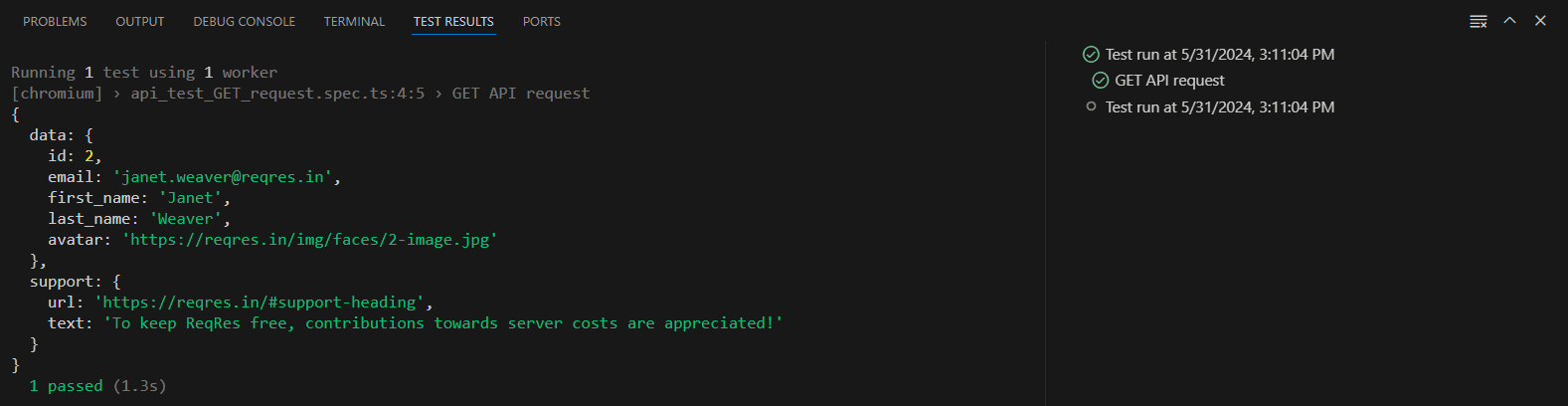
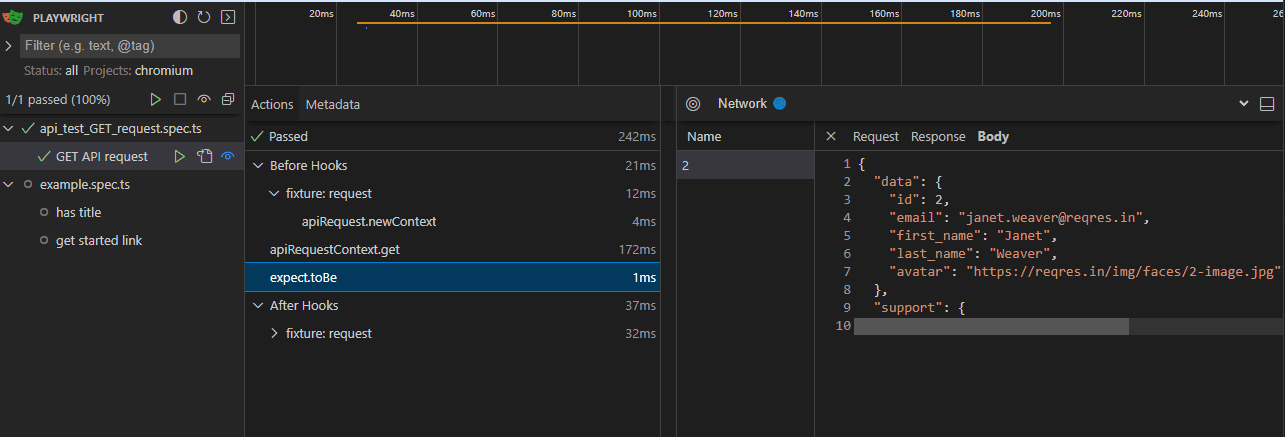
↪️To create a test file and perform a POST request using Playwright, follow these detailed steps:
For performing a POST request, you have the flexibility to create a new test file or include it in an existing test file. Both approaches are valid and can be chosen based on your project structure and organization preferences.
import {test, expect} from '@playwright/test';
import { request } from 'http';
import { text } from 'stream/consumers';
test('POST API request',async({request}) =>{
const response = await request.post('https://reqres.in/api/users',{
data:{
"name": "morpheus",
"job": "leader"
}
})
expect(response.status()).toBe(201)
console.log(await response.json());
})
Above code performs an asynchronous POST request to the specified URL (https://reqres.in/api/users) using the Playwright request object. The await keyword ensures that the code waits for the request to complete before proceeding. The second argument is an object containing the request payload (data), which includes the name and job properties. The response from the API call is stored in the response variable.
expect(response.status()).toBe(201);Above code line uses the expect function to assert that the status code of the response is 201, which indicates that a new resource was successfully created.
Run the Test Results:

Conclusion:
In conclusion, using Playwright with TypeScript for performing API requests offers a powerful and efficient way to automate testing. Playwright provides a straightforward interface for making HTTP requests, allowing developers to easily interact with APIs and validate responses within their test suites. TypeScript’s strong typing capabilities enhance code readability and maintainability, making it easier to catch errors and write more robust tests. Playwright, while powerful, does have some limitations. It offers limited support for non-browser protocols, as it can only handle HTTP/HTTPS and browser-specific data. Additionally, Playwright does not support browser extensions, which can be a drawback for certain testing scenarios. The framework can also struggle with pages containing dynamic content or heavy JavaScript, potentially leading to inconsistencies in test results. Furthermore, Playwright has limited support for mobile browsers, which may affect its effectiveness in testing mobile web applications. Lastly, some browser-specific features, such as reader mode or reading list, might not work correctly with Playwright.
Overall, leveraging Playwright with TypeScript enables developers to streamline their API testing process, leading to more reliable and maintainable test suites.
Keep practicing and exploring to master these powerful tools further with Jignect.
Witness how our meticulous approach and cutting-edge solutions elevated quality and performance to new heights. Begin your journey into the world of software testing excellence. To know more refer to Tools & Technologies & QA Services.
If you would like to learn more about the awesome services we provide, be sure to reach out.
Happy Testing 🙂