Testing is super important in making sure the software works well. Test Automation makes this easier by using special tools and programs to do tests quickly and accurately. This helps check if the software does what it’s supposed to do, how well it performs, and if it’s reliable.
By automating repetitive testing tasks, teams can significantly expedite their testing procedures, expand test coverage, and elevate the overall quality of their software products. Notably, Test Automation assumes a pivotal role in contemporary software development methodologies like Agile and DevOps, empowering teams to deliver superior-quality software products in a more streamlined and efficient manner.
What is Playwright?
- The playwright is the most recent Test Automation tool in the software testing industry. It is developed and supported by Microsoft and is one of the best tools for UI and API testing. The playwright does not require any complicated configuration. Everything like – Typescript, assertion library, Test runner, etc. are packed by default inside Playwright.
- People who use Playwright can handle web browsers such as Chrome, Edge, Firefox, and Safari just like they do with their mobile phones when taking pictures, recording videos, or engaging with others on social media.
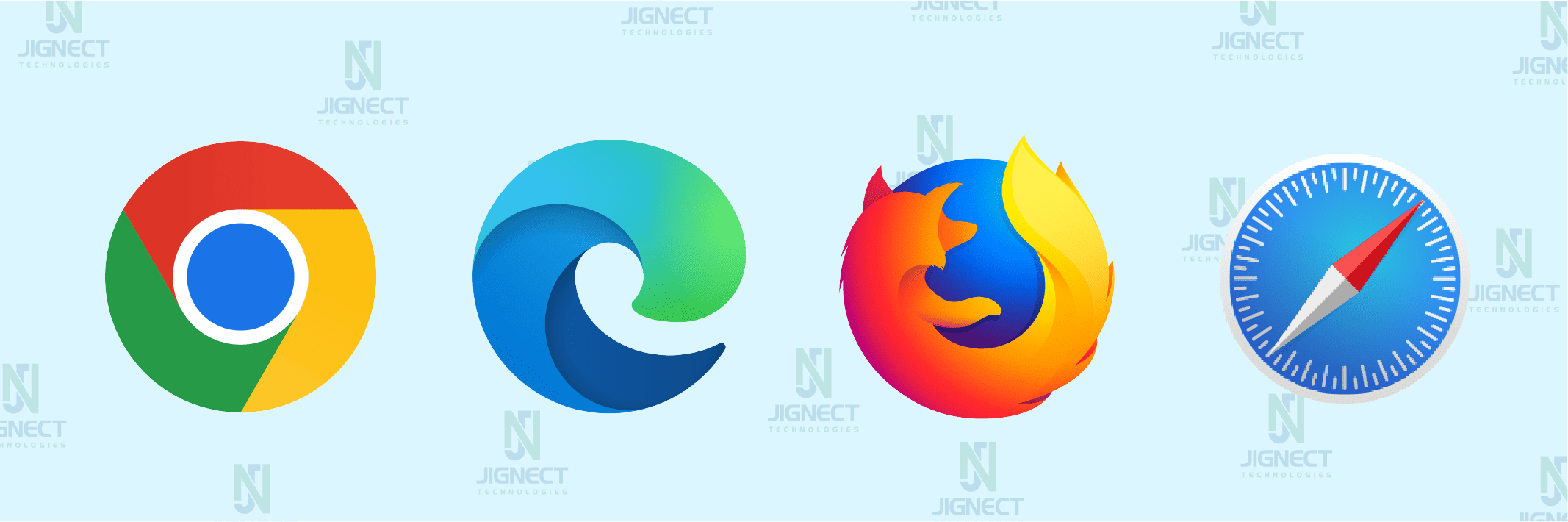
- The official documentation for Playwright can be found at https://playwright.dev/
What is TypeScript?
- TypeScript is a superset of JavaScript that adds static typing to the language. Playwright is a tool for automating browser actions, such as clicking buttons, filling forms, and navigating between pages. TypeScript provides type checking and other advanced features that can improve the development experience when working with Playwright.
Why PlayWright with TypeScript?
- Combining Playwright with TypeScript offers a powerful solution for web application testing. Playwright enables automated browser testing, allowing you to simulate user interactions across various browsers. TypeScript adds static typing and advanced features to JavaScript, enhancing code quality and maintainability.
Main Features & Capabilities of Playwright:
1. Automated Browser Testing: Playwright simplifies end-to-end testing by offering a high-level API to interact with web browsers.
2. Type Safety: TypeScript’s static typing ensures code correctness, catching errors early in development and improving code maintainability.
3. Test Frameworks: Popular frameworks like Jest, Mocha, or Jasmine can be used with Playwright and TypeScript to define test cases and assert expected outcomes.
4. Page Object Model (POM): Implementing POM allows encapsulating web pages as objects, making tests modular and easier to maintain.
5. Continuous Integration (CI): Integrate Playwright tests into CI pipelines for automated testing upon code changes, ensuring early detection of regressions.
6. Reporting and Analysis: Playwright supports generating detailed test reports for analysis, aiding in identifying and diagnosing issues.
7. Auto-Wait: Playwright automatically waits for elements to be actionable before performing actions, eliminating the need for artificial timeouts and reducing test flakiness.
8. Browser Contexts: Playwright creates a browser context for each test, equivalent to a brand-new browser profile, delivering full test isolation with zero overhead. Creating a new browser context is fast, taking only a few milliseconds.
9. Tracing and Debugging: Playwright provides built-in tracing capabilities to capture execution traces, videos, and screenshots during test runs, aiding in identifying and debugging issues and eliminating flaky tests.
10. Support for Shadow DOM and Frames: Playwright’s selectors can pierce through Shadow DOM and seamlessly enter frames, allowing interaction with elements within these contexts during testing.
11. Cross-Language Support: Playwright supports multiple programming languages, including TypeScript, JavaScript, Python, Java, and .NET (C#), enabling teams with diverse language preferences to collaborate and contribute to testing efforts using their preferred language.
The recommended IDE for working with Playwright is Visual Studio Code (VS Code). VS. Code is a popular and the lightweight code editor that provides excellent support for TypeScript, JavaScript, and debugging capabilities. It offers a seamless development experience for Playwright, allowing you to write, debug, and test your Playwright scripts efficiently.
However, it’s worth noting that Playwright is a versatile framework, and you can use other IDEs as well, depending on your preferences. Some developers also use JetBrains IDEs like IntelliJ IDEA or WebStorm for Playwright development. These IDEs offer features like code completion, debugging, and integrated terminal support.
Prerequisite Steps
In this blog, during the practical demonstration, we utilized the following versions for the respective libraries and applications:
- Playwright version: 1.42.1
- VS code version: 1.87.2
- Node JS version: v18.18.1
Download & Install VS code:
- To download the VS code, visit its official website and choose the .exe file for the community version.
- After the download finishes, launch the .exe file and proceed with installing VS Code.
- You can refer to the instructions provided on this website for guidance on Installing VS code.
Installing Node On Windows:
- Downloading the Node.js ‘. MSI’ installer the first step to installing Node.js on Windows is to download the installer. Visit the official Node.js website i.e) https://nodejs.org/en/download/
- Running the Node.js installer.
- Double-click on the .msi installer.
- Select “Next” up to the Finish button.
- Verify that Node.js was properly installed or not using the “C:\Users\Admin> node -v” command. If node.js was completely installed on your system, the command prompt will print the version of the Node JS installed.
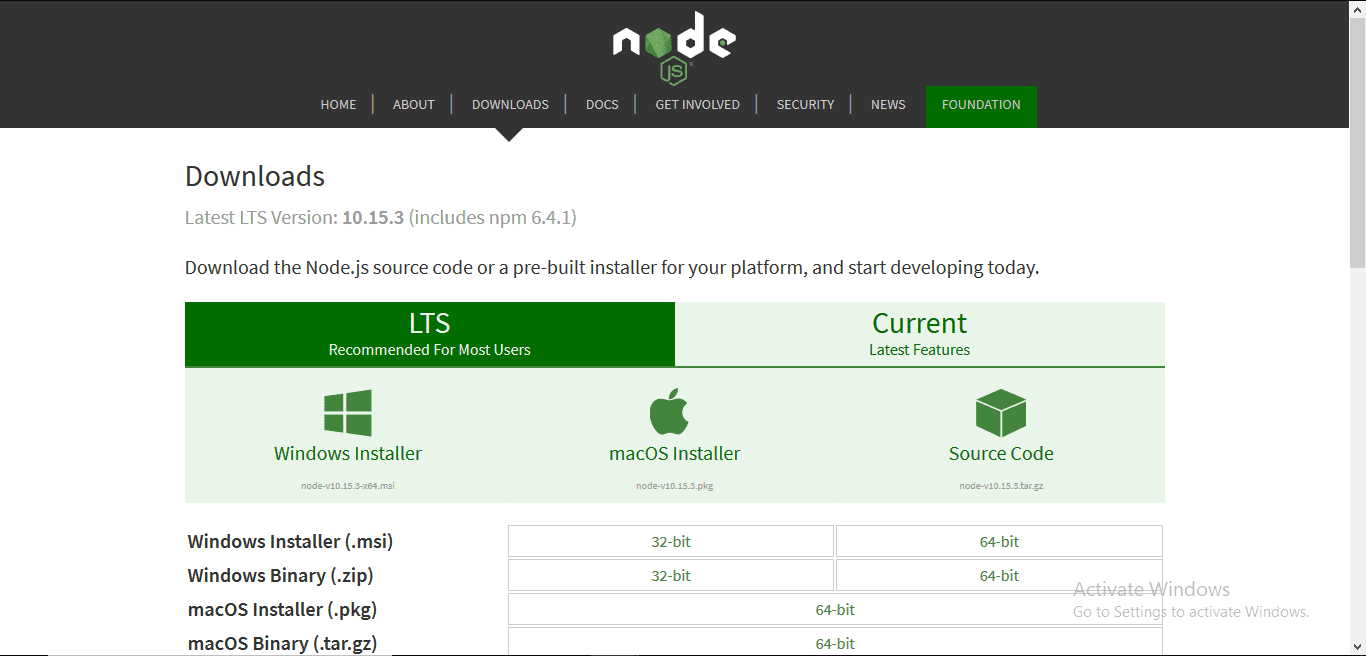
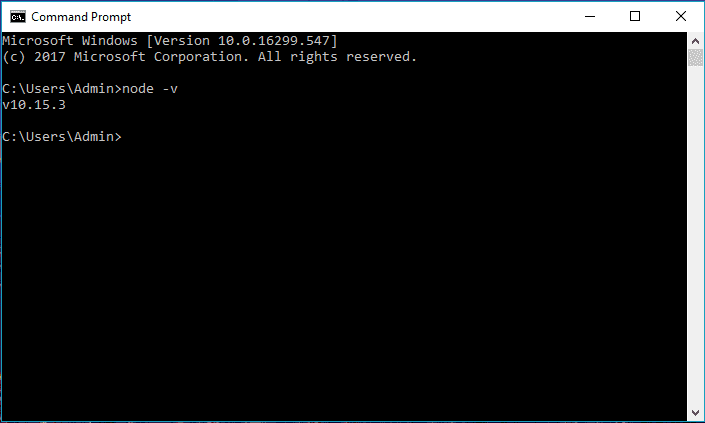
Installing playwright:
There are 2 ways to install a playwright.
1. Using Command Line
- npm init playwright@latest
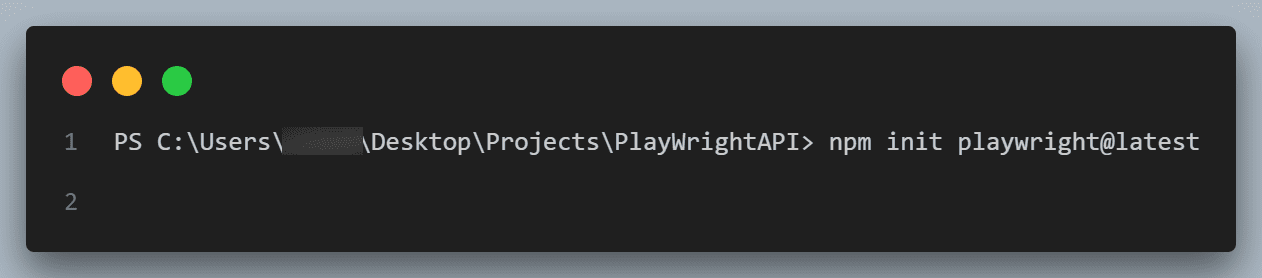
2. Using the Extension
- Open Your Code Editor: Launch your preferred code editor. For example, if you’re using Visual Studio Code (VS Code), open it on your system.
- New Project: Create a new project and open it into the vs code.
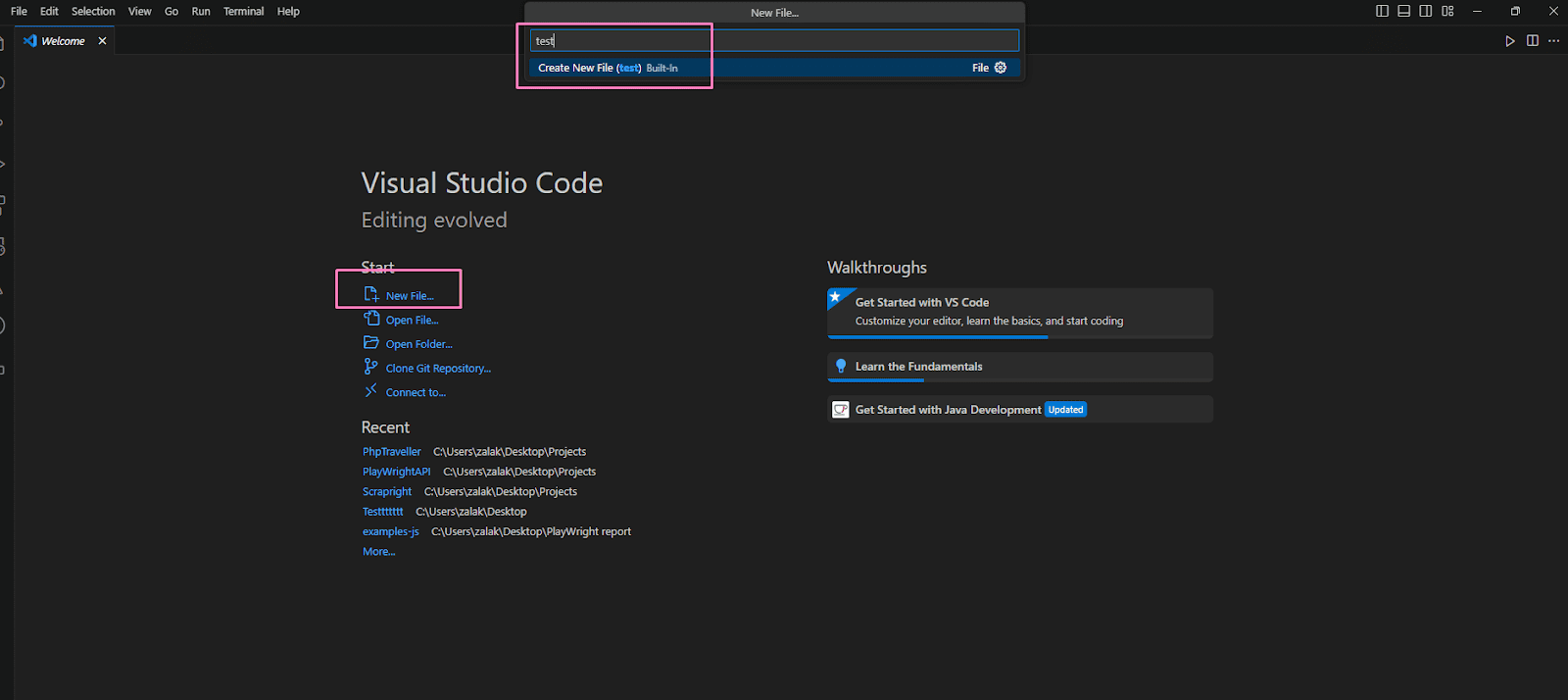
- Navigate to Extensions Marketplace: Look for the extensions marketplace within your code editor. In VS Code, you can find it on the left sidebar or by pressing Ctrl+Shift+X.
- Search for Playwright Extension: In the extensions marketplace, search for the Playwright extension. You can find it by typing “Playwright” into the search bar.
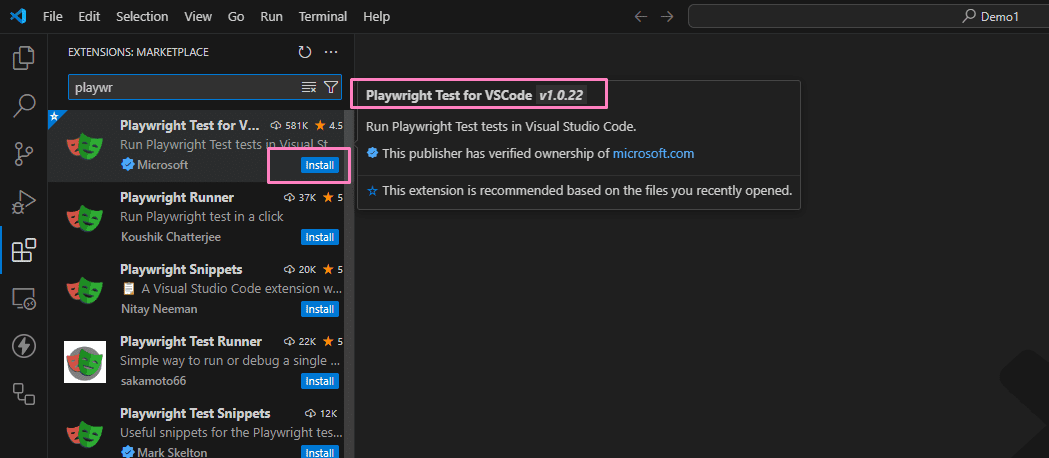
- Install the Extension: Once you’ve found the Playwright extension, click on the “Install” button next to it. Wait for the installation process to complete.
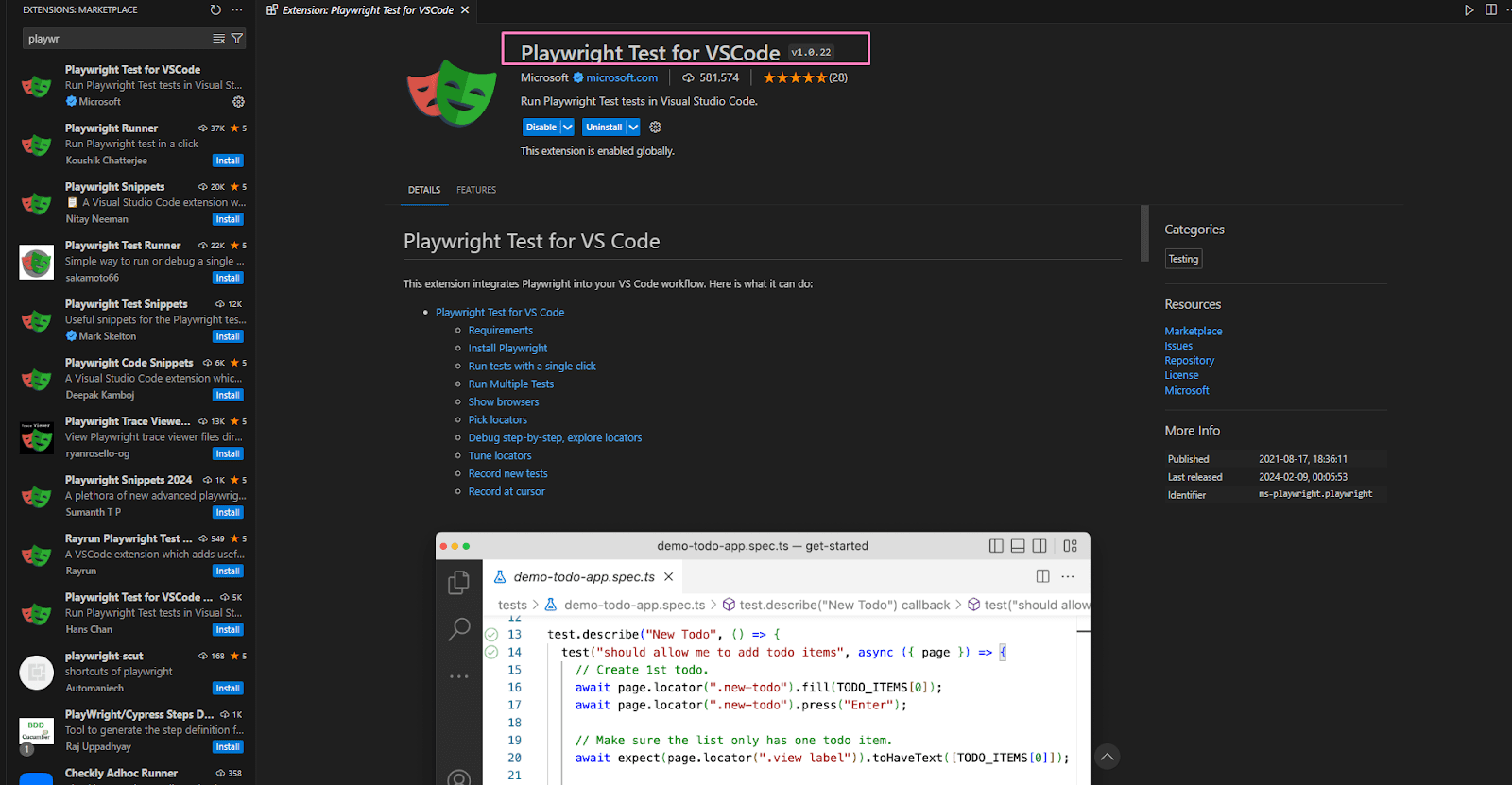
- Install the Playwright: Press Ctrl + shift + P and search the `Playwright` keyword in the search box. Click on the `Install playwright` button and also install the necessary browser.
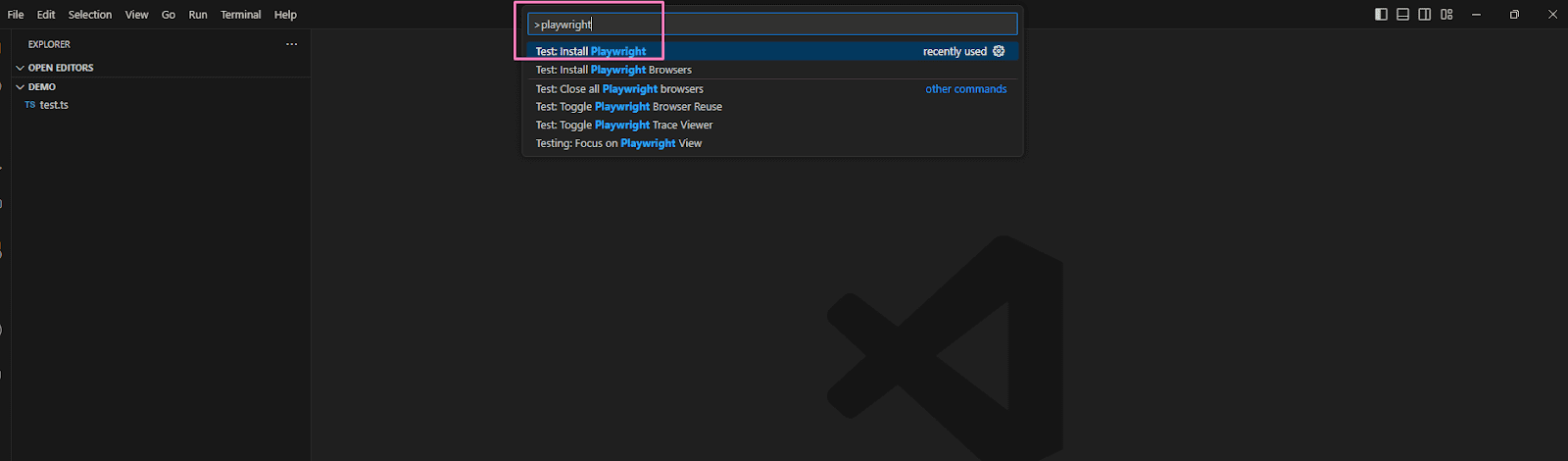
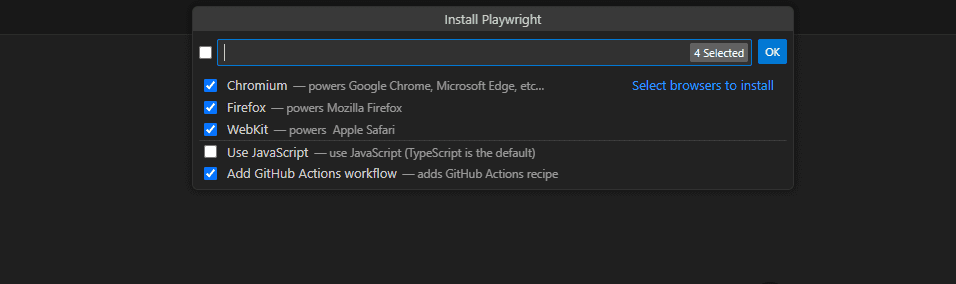
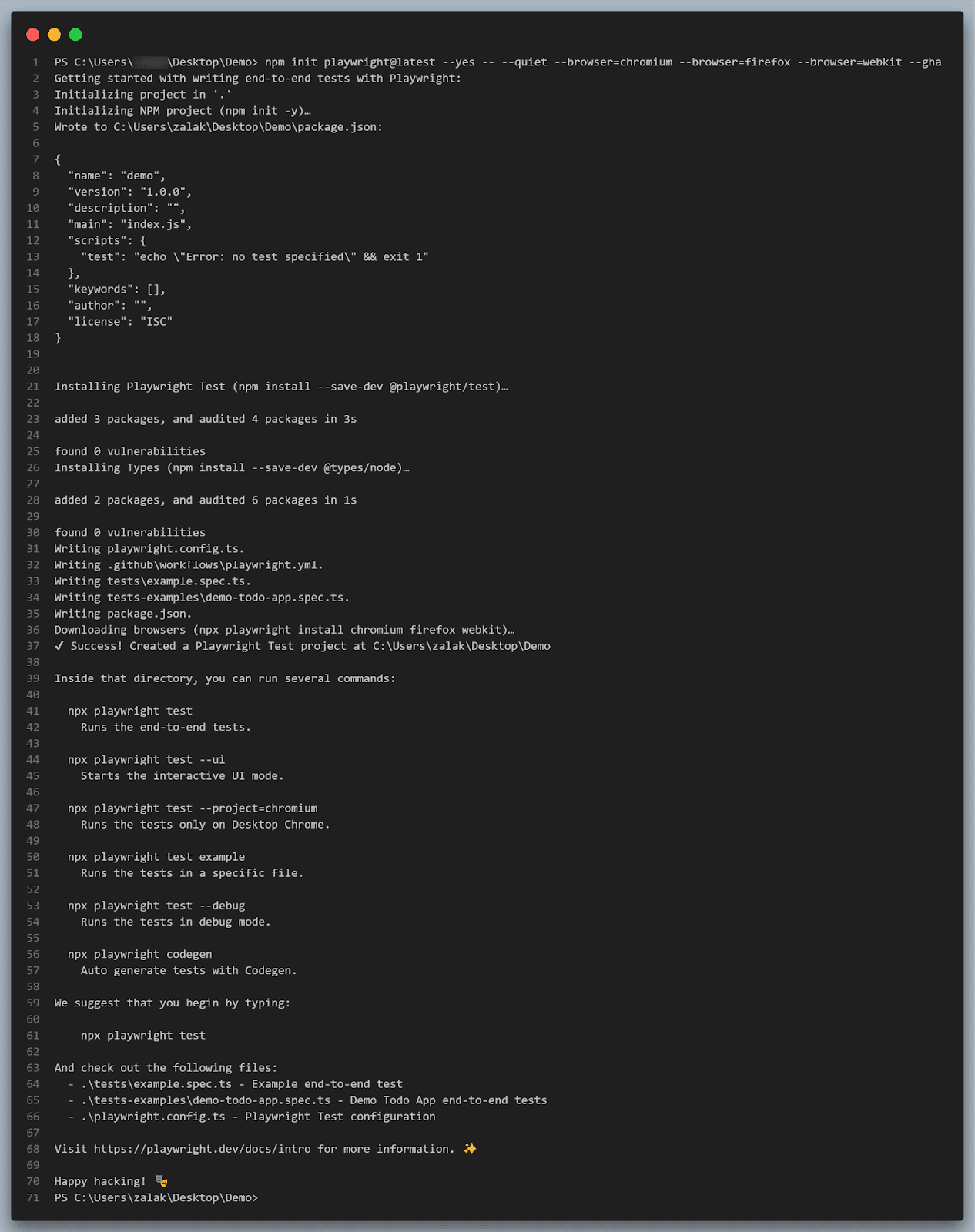
Test Case Creation
- For writing the first script, we first need to create a javascript or typescript file.
- In this typescript, the file extension must be a .ts
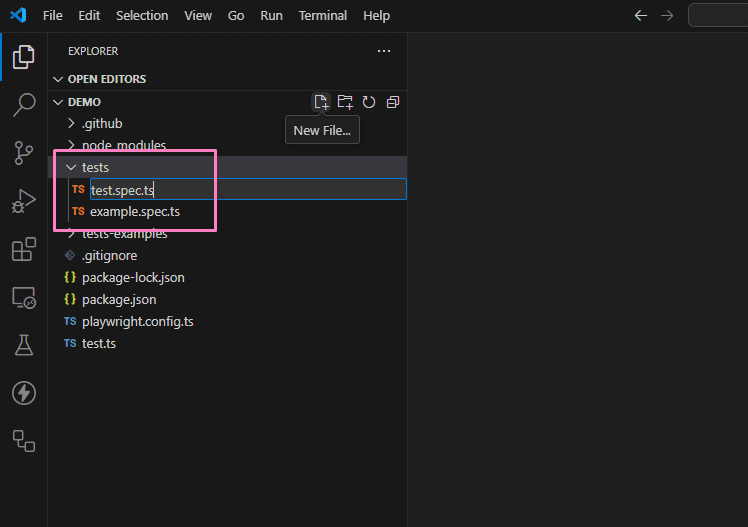
- We can add the below code to the file.
import test, { expect } from "@playwright/test";
import { chromium } from "playwright";
test.describe("Navigation Test", () => {
test("Verify that the user is navigate on the `Life At Jignect` page successfully", async () => {
//Page and browser
var browser = await chromium.launch();
var context = await browser.newContext();
var page = await context.newPage();
//Navigate to the URL
await page.goto(`https://jignect.tech/`);
//Locators
const menuText = page.locator(`//div[@id='site-header']//a[text()='About Us']`);
const subMenuText = page.locator(`//div[@id='site-header']//a[text()='Life at Jignect']`);
const headingText = page.locator(`//div[contains(@class,'heading')]`);
//Actions
await menuText.hover();
await subMenuText.click();
const titleText = await headingText.textContent();
//Assertion
expect(titleText).toBe('Life At Jignect');
});
});
- Let’s understand the example outlined above.
- Imports:
- The code starts by importing `test` and `expect` from @playwright/test. These are used for writing tests and assertions, respectively.
- It also imports `chromium` from `playwright` which is a browser engine that Playwright can control.
- Test Description:
- `test.describe()` function defines a test suite named “Navigation Test”. A test suite is a collection of test cases that test a specific feature or functionality.
- Test Function:
- Inside the test suite, there’s a test function defined using test() function. This test function verifies that the user can successfully navigate to the “Life At Jignect” page.
- The test function is defined using an async function because it contains asynchronous operations (e.g., launching browser, navigating to URL, interacting with elements).
- Page Setup:
- Inside the test function, a Chromium browser instance is launched using `chromium.launch()`.
- A new browser context is created using `browser.newContext()`.
- A new page is opened in the browser context using `context.newPage()`.
- Assertion:
- Finally, an assertion is made using `expect()` function to verify that the `titleText` retrieved from the heading element is equal to ‘Life At Jignect’.
- Imports:
Run the created Test Case and check the Result
1. Once you’ve written the test script, run the test and check the results carefully.
There are two ways to run the test script.
- Open the terminal and hit the command: npx playwright test {testname}.
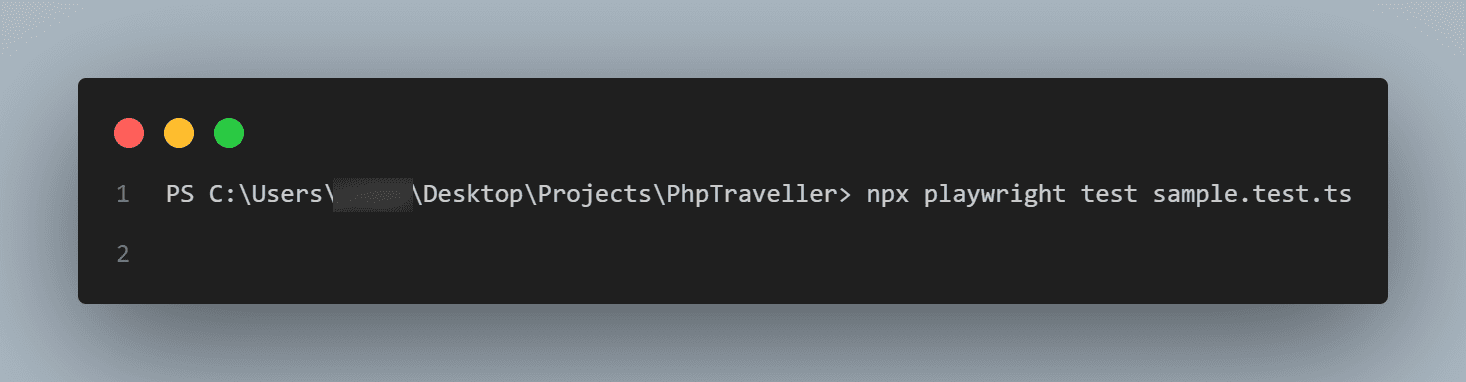
- Another way is by clicking on the green triangle from the code.
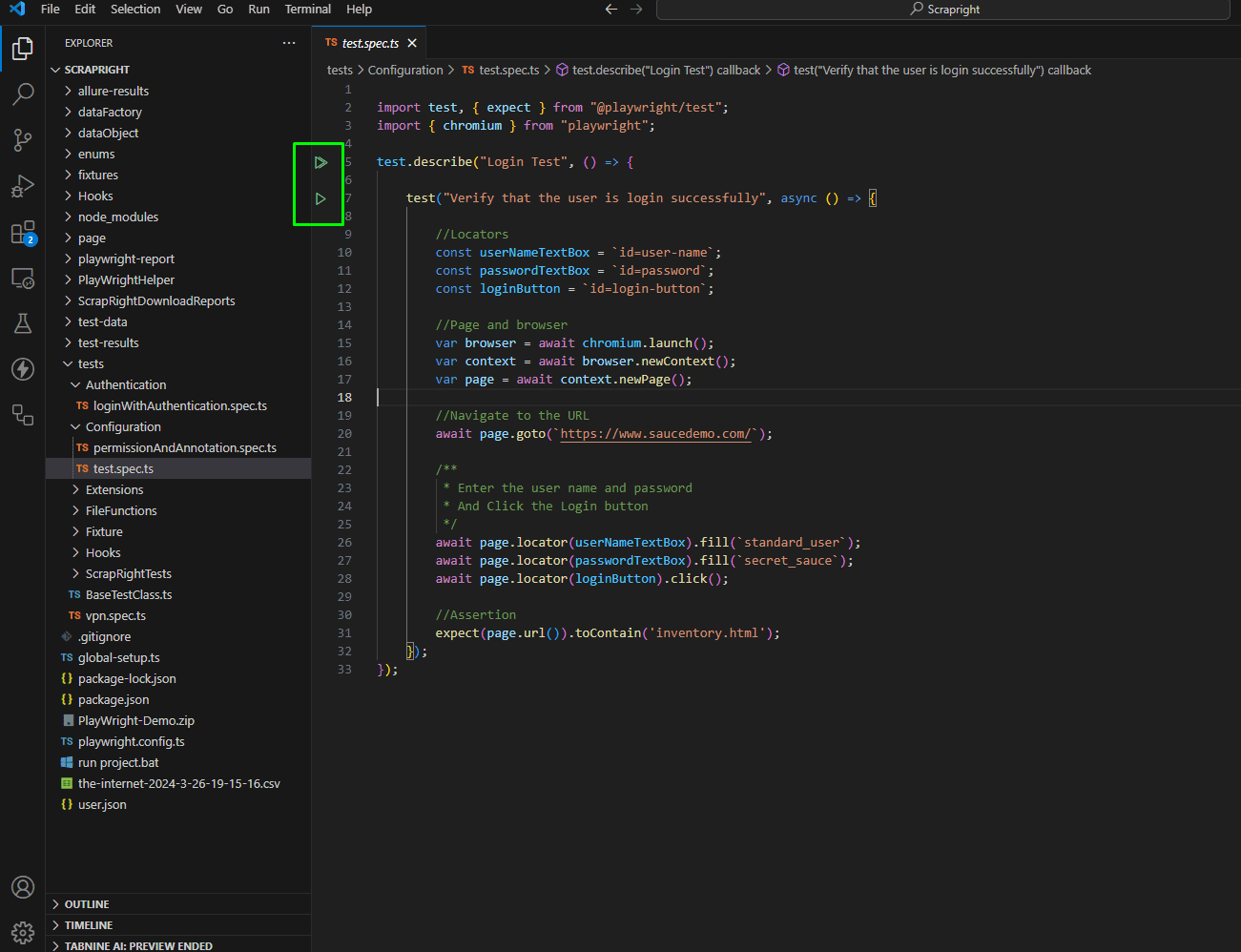
2. Test Run Result
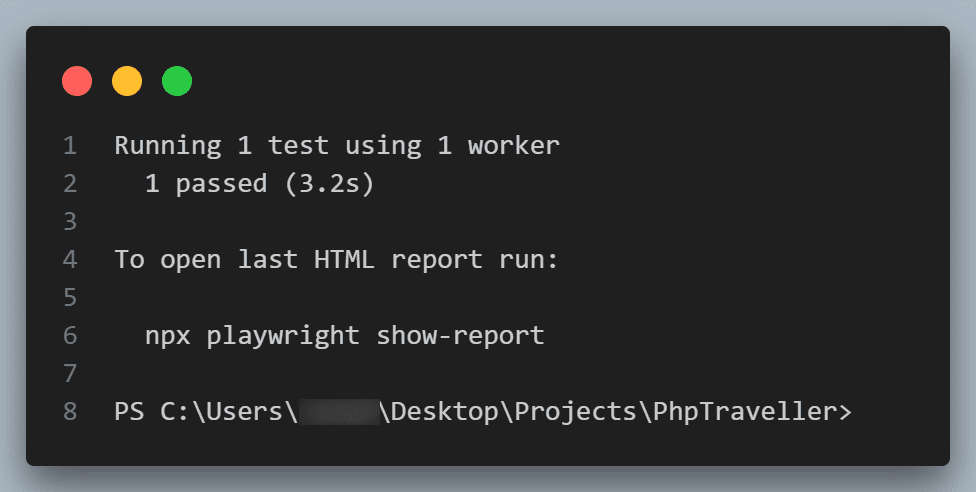
Conclusion
In summary, the integration of Playwright with TypeScript offers a robust and efficient solution for web application testing. Playwright simplifies browser automation with its high-level API and comprehensive features, while TypeScript enhances code quality and maintainability with static typing and advanced language features. Together, they empower development teams to accelerate testing processes, improve test coverage, and deliver superior-quality software products in a streamlined and efficient manner. By leveraging Playwright and TypeScript, teams can achieve their testing objectives effectively and confidently, ensuring the reliability and performance of their web applications.
However, it’s important to note some limitations of the Playwright:
- Full-screen functionality is not supported.
- Using multiple locators simultaneously is not supported.
Witness how our meticulous approach and cutting-edge solutions elevated quality and performance to new heights. Begin your journey into the world of software testing excellence. To know more refer to Tools & Technologies & QA Services.
If you would like to learn more about the awesome services we provide, be sure to reach out.
Happy Testing 🙂