In software testing and QA, ensuring that your codebase remains organized, reliable, and traceable is essential. This is where Git, a widely-used version control system, proves to be incredibly valuable. For QA teams, Git helps streamline the management of test scripts, track changes efficiently, and ensure that testing environments stay intact.
With Git, software testers can monitor the entire history of their test automation scripts. This makes it easier to pinpoint when a bug was introduced and understand why certain changes were made. Moreover, if needed, you can easily revert to an earlier version of your codebase. Git also promotes seamless collaboration between developers and testers by allowing code and test script changes to be reviewed, merged, or rolled back in a controlled way.
When running automated test scripts, managing test data, or adjusting configurations, Git makes the entire process more efficient. It’s not just for code developers; testers benefit from its capabilities just as much.
One of the standout features of Git for QA teams is its branching system. You can create separate branches for different types of tests, like regression or performance testing, without affecting the main version of your code. These branches allow QA Automation Engineers to run isolated tests, fix issues, and then merge everything back into the main branch once the tests are successful. This process keeps your testing clean, structured, and well-organized.
To gain a deeper understanding of Git and explore its features, check out this blog: Git Workflow
- Basic Git Command
- git init – Initialize a Repository
- git clone – Clone an Existing Repository
- git add – Stage Changes for Commit
- git branch – Manage Branches
- git commit – Commit Changes
- git push – Push Changes to Remote Repository
- git pull – Fetch and Merge Updates from Remote
- git status – Check the Current Status
- git merge – Merge Branches
- Advanced Git Commands
- Best Practices for Using Git
- Conclusion
Basic Git Command
git init – Initialize a Repository
- This command initializes a new Git repository in your project directory. It creates the .git folder that Git uses to track changes.
- git init
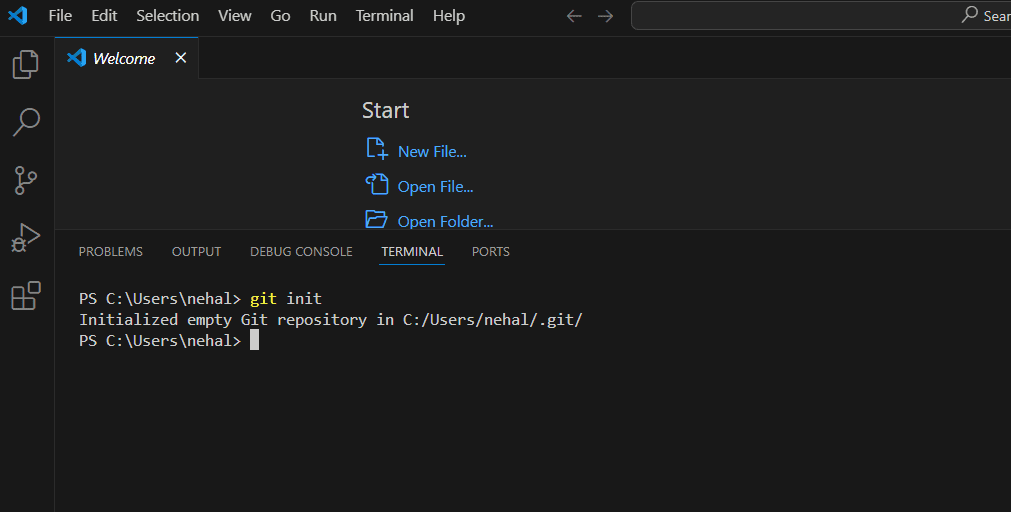
git clone – Clone an Existing Repository
- Used to copy an existing repository to your local machine. This command downloads all files and commits from a remote repository.
- git clone <repository-url>
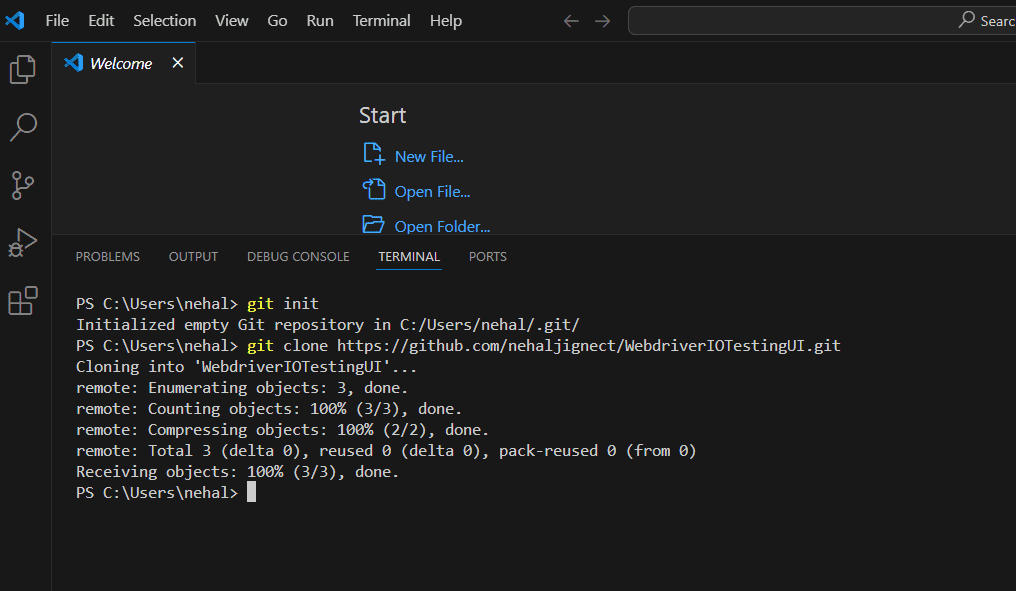
git add – Stage Changes for Commit
- This command stages files for commit. You can add specific files or directories or use git add . to stage everything.
- git add test.e2e.js
Git add specific file.
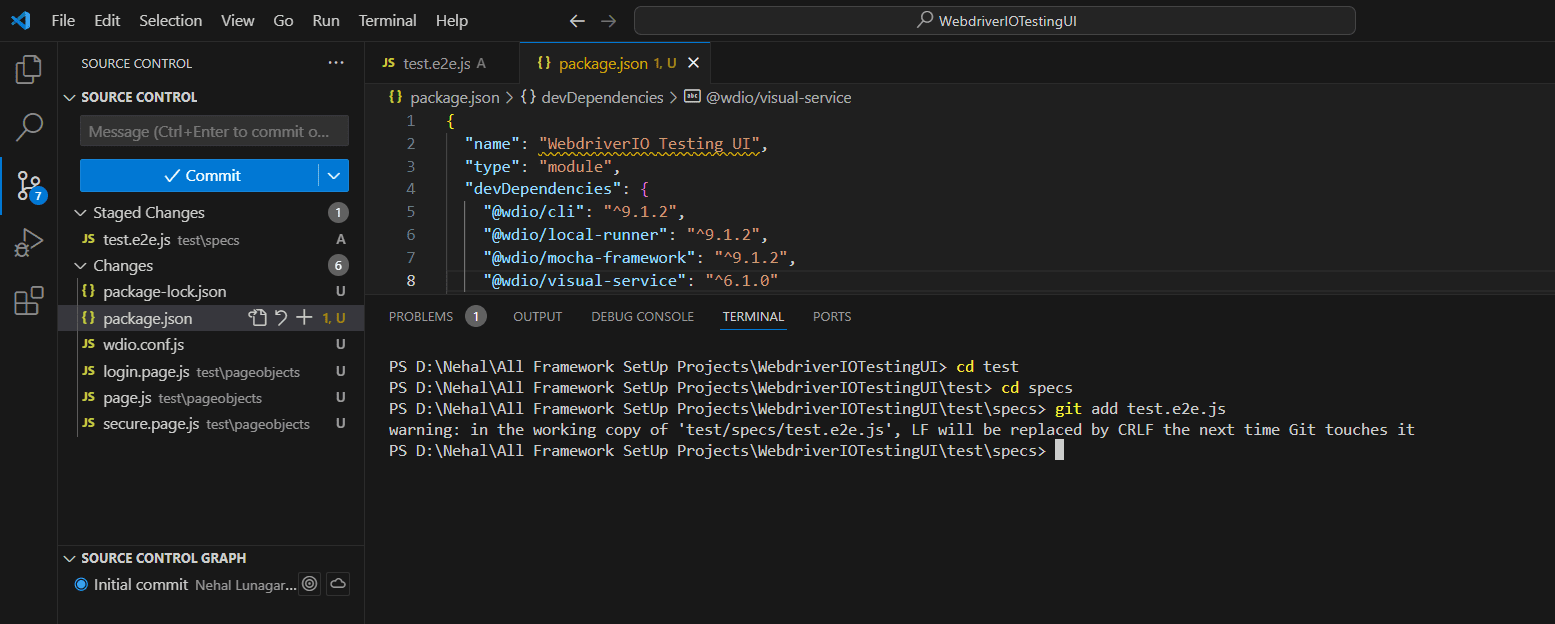
Git add all files.
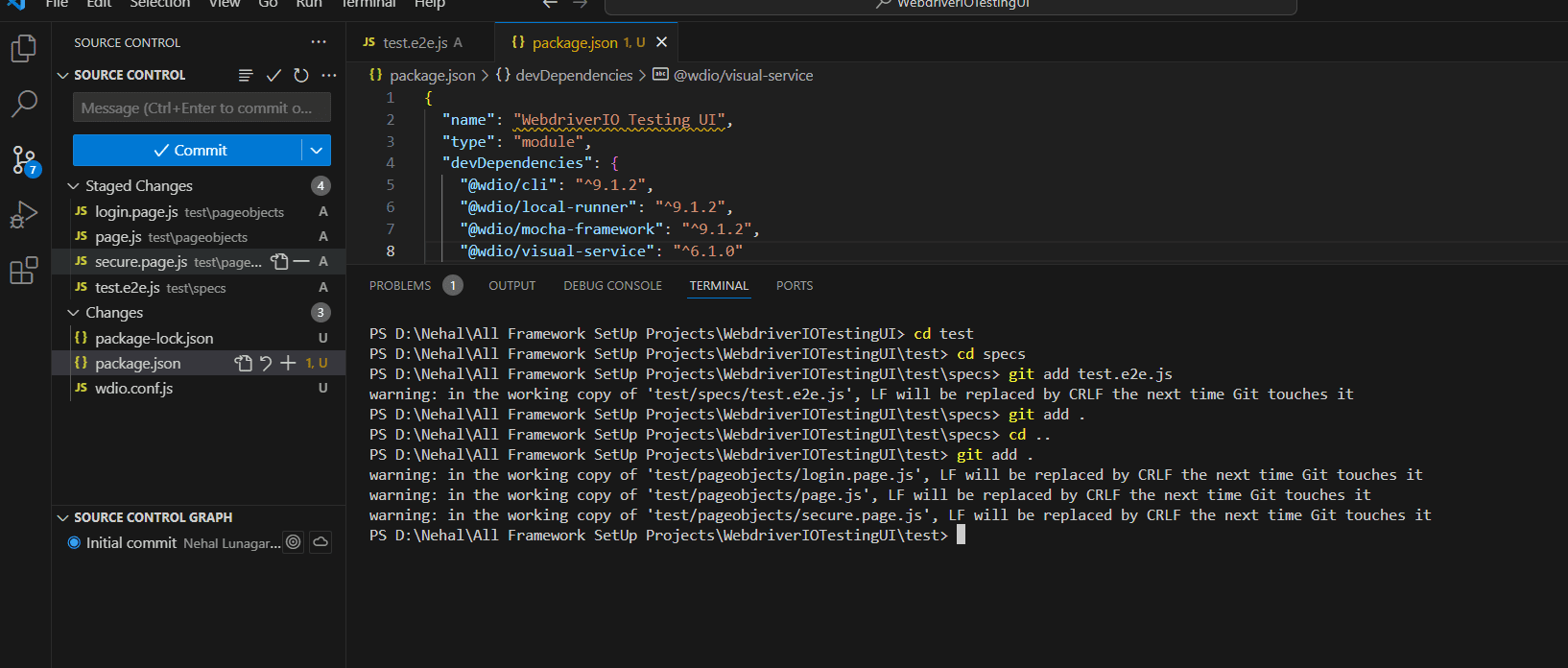
git branch – Manage Branches
- Lists, creates, or deletes branches in the repository.
- git branch <branch-name> # Create a new branch git
- branch -d <branch-name> # Delete a branch
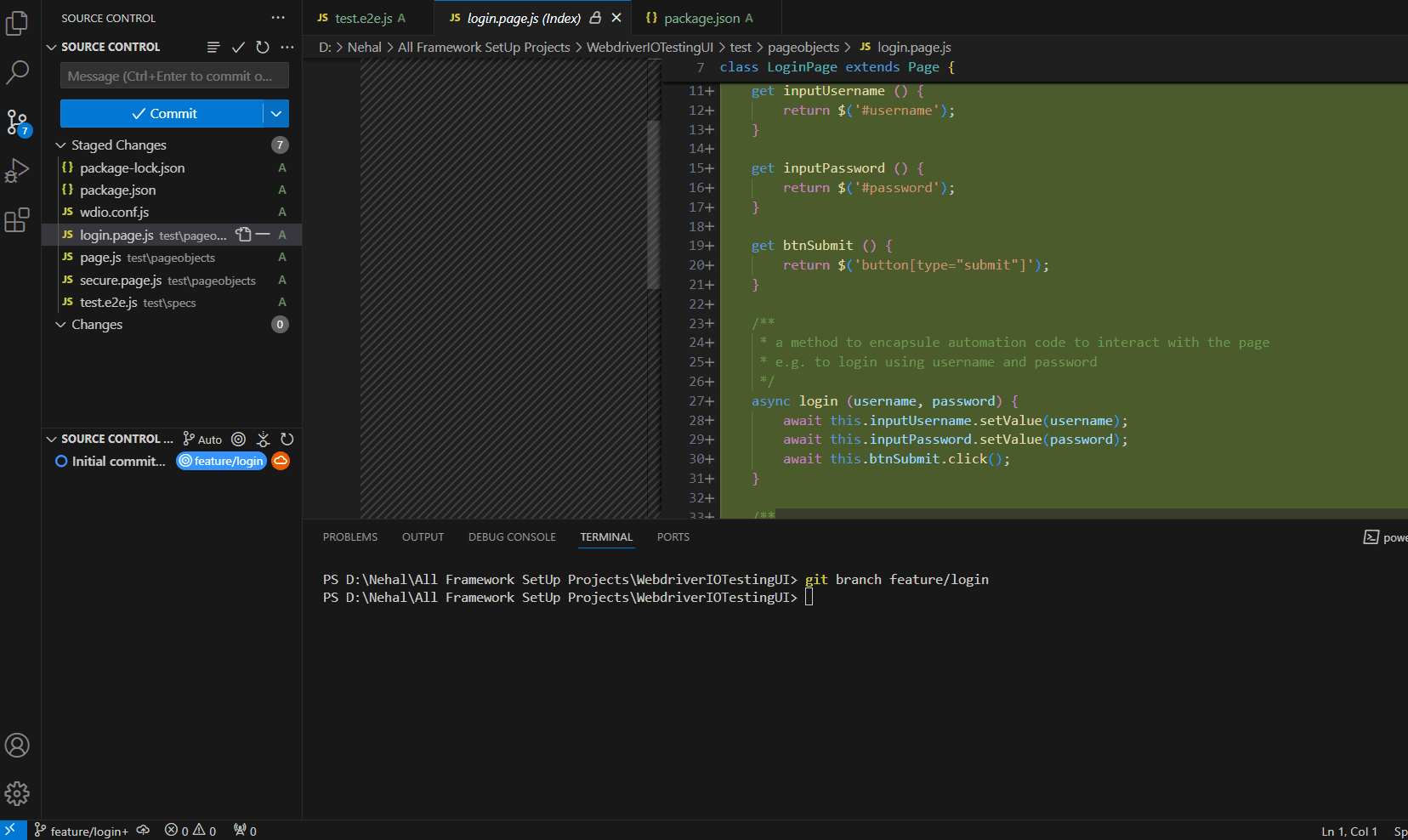
git commit – Commit Changes
- The git commit command saves your changes to the repository with a descriptive message.
- git commit -m “Commit message”
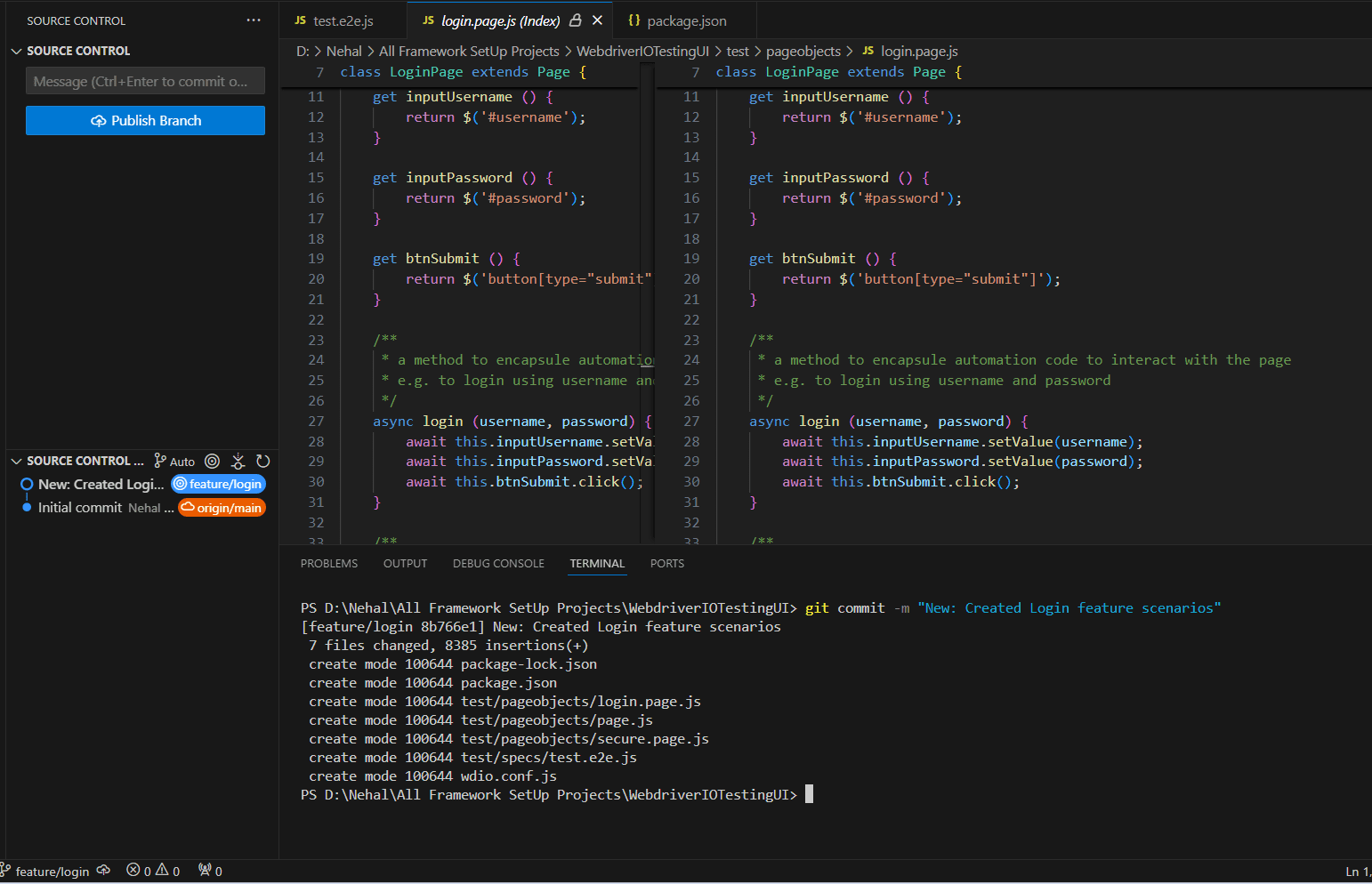
git push – Push Changes to Remote Repository
- Once you’ve committed changes, you can push them to a remote repository.
- git push origin <branch-name>
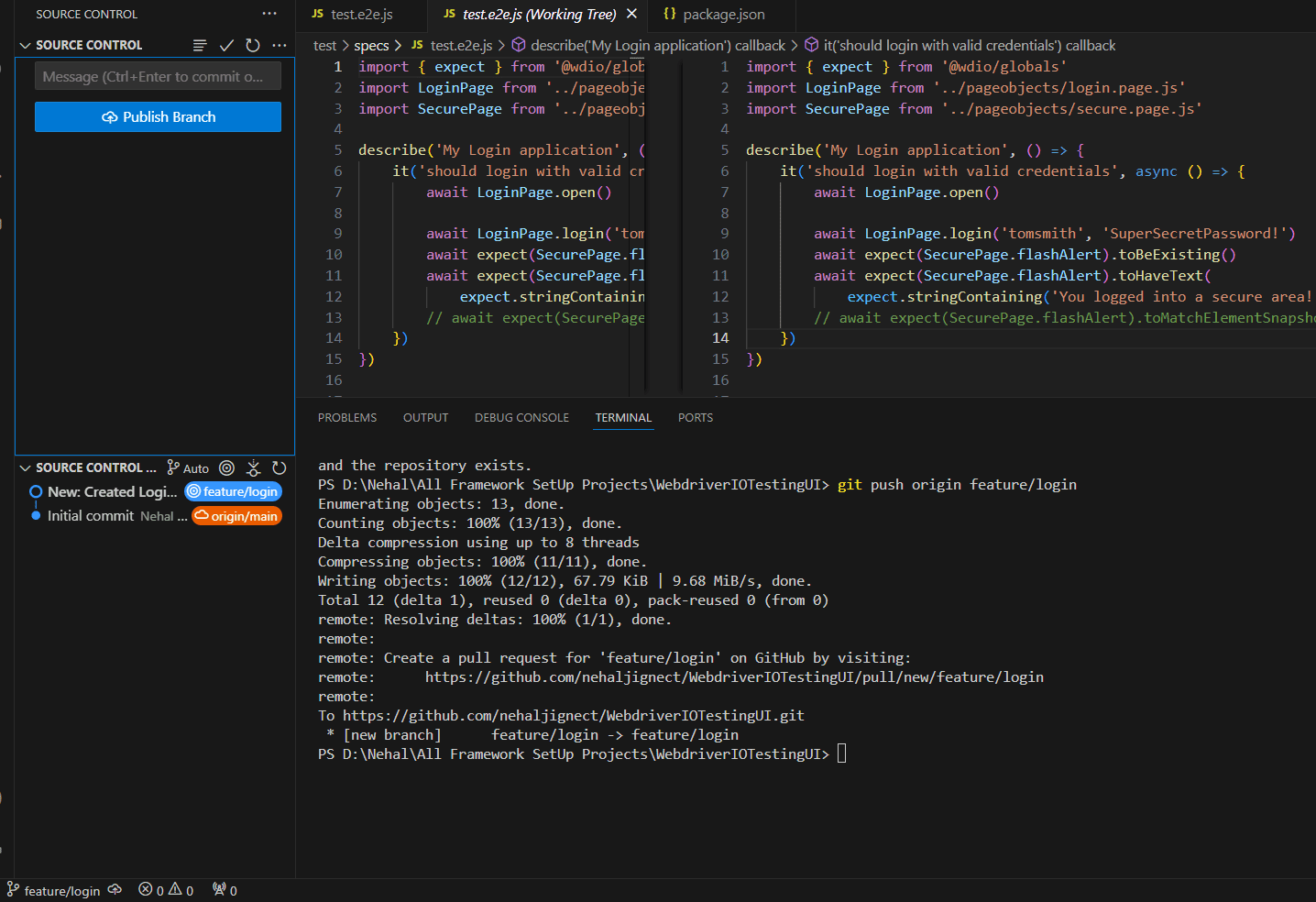
git pull – Fetch and Merge Updates from Remote
- Fetches and merges changes from a remote repository into your current branch.
- git pull origin <branch-name>
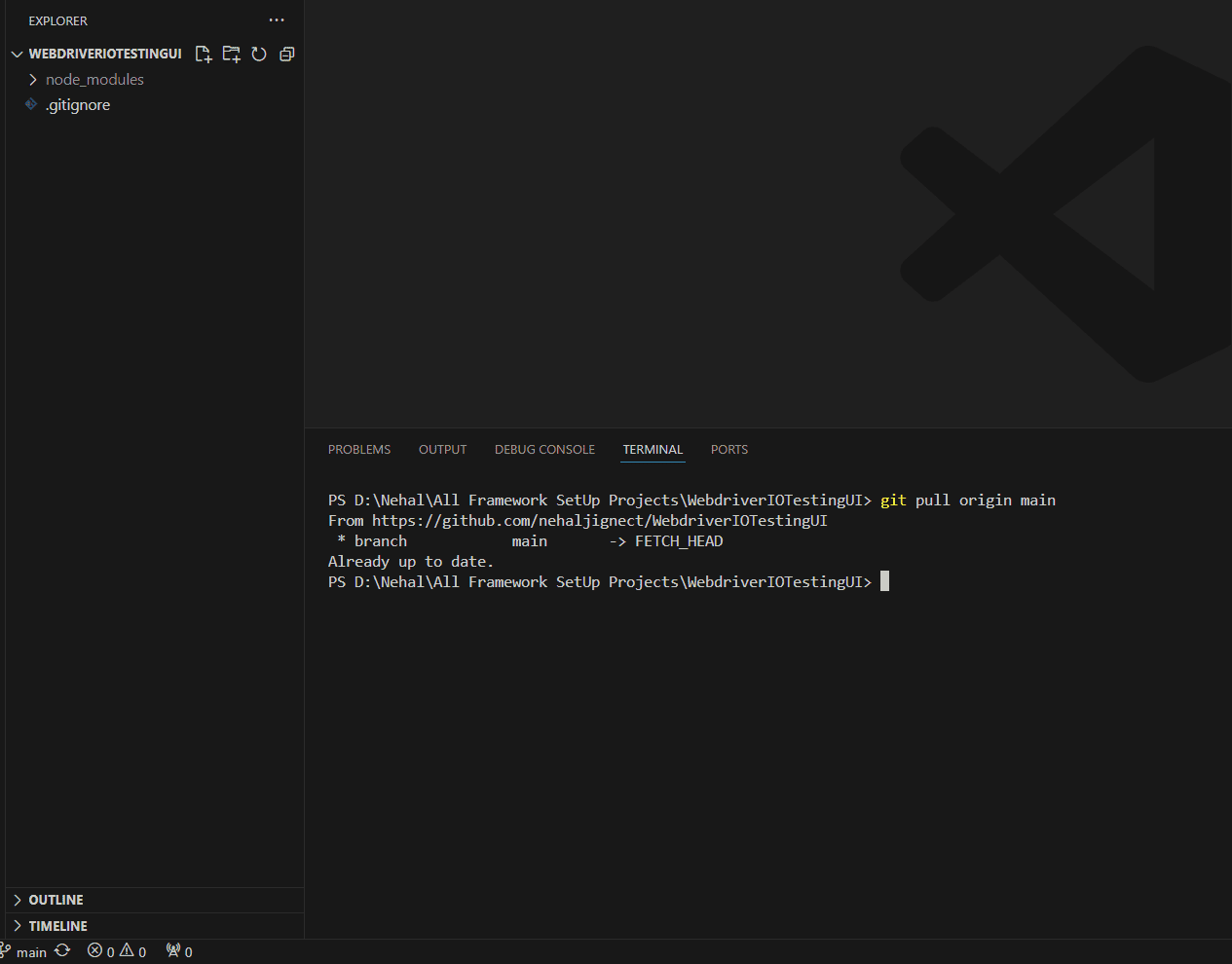
git status – Check the Current Status
- Displays the state of the working directory and staging area. It shows which changes have been staged and which haven’t.
- git status
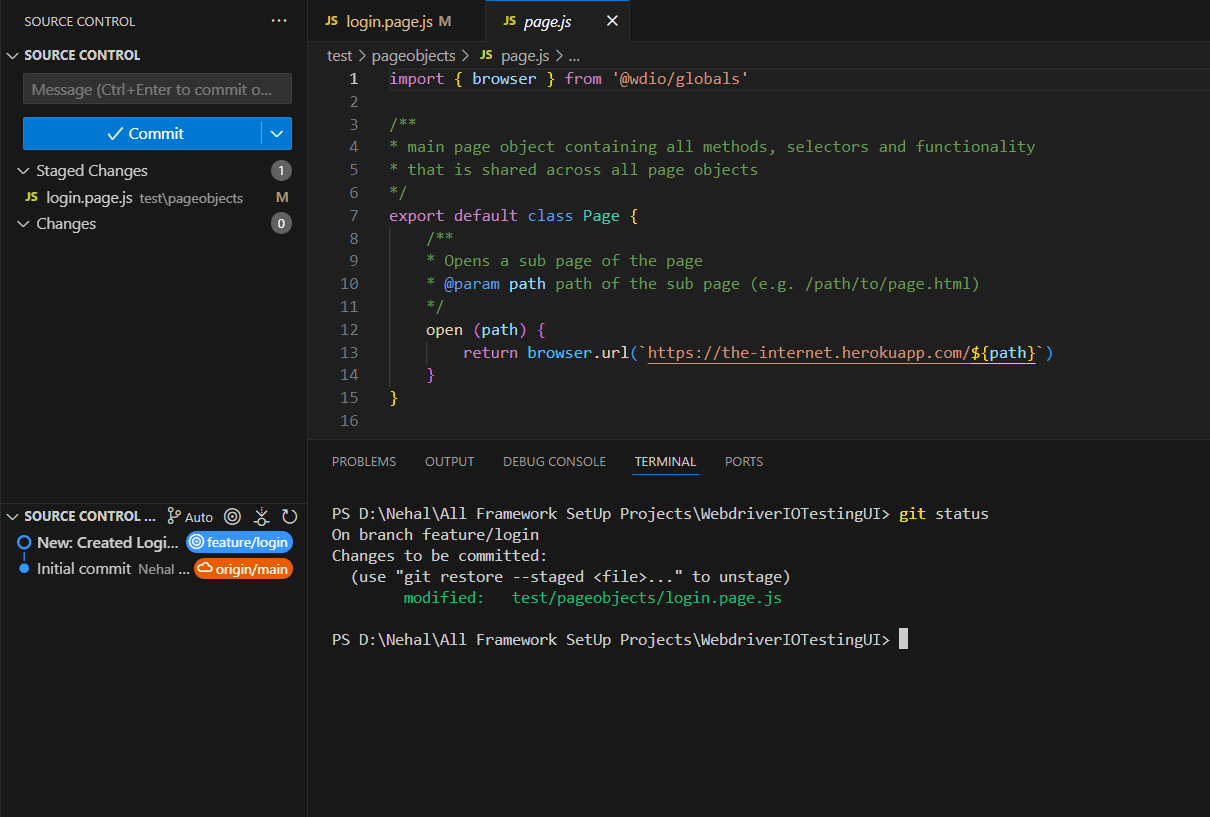
git merge – Merge Branches
- Merges another branch into the current branch.
- git merge <branch-name>
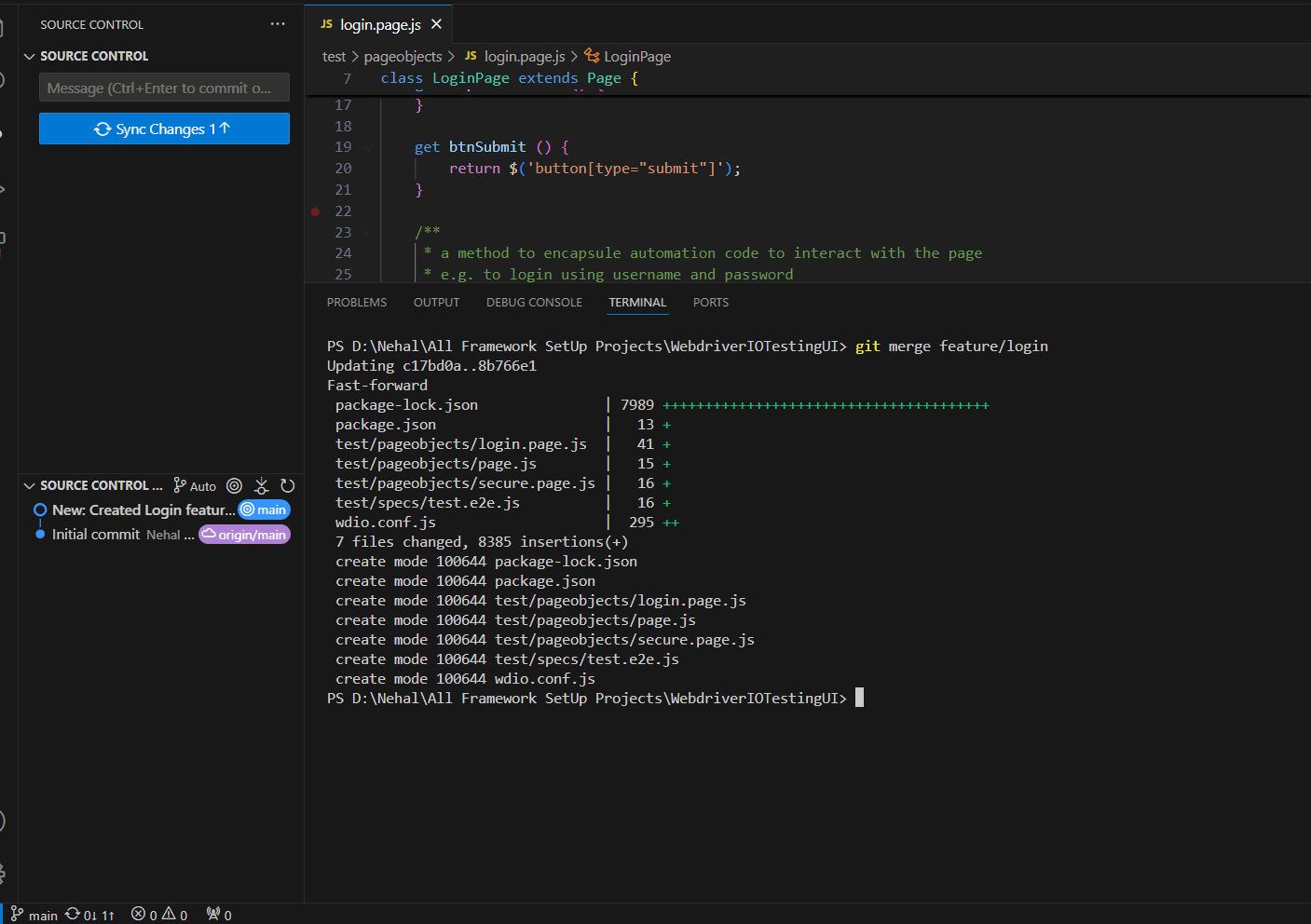
Advanced Git Commands
- git stash – Temporarily Save Changes
- Temporarily save your changes without committing them. This is useful when you need to switch branches without losing your current work.
- git stash
- git stash pop # To retrieve the changes
- git rebase – Keep Commit History Clean
- The git rebase command integrates changes from one branch into another. It helps maintain a clean project history without merge commits.
- git rebase <branch-name>
- git cherry-pick – Apply Specific Commits
- This command allows you to apply specific commits from one branch to another.
- git cherry-pick <commit-hash>
- git reset – Undo Changes
- Undo changes in the working directory or the repository. Use git reset –soft to keep changes staged, or git reset –hard to discard them.
- git reset –hard <commit-hash>
- git reflog – View Every Action
- git reflog helps track all the changes you’ve made in Git, even those that aren’t visible through the regular log.
- git reflog
- git bisect – Identify the Commit That Introduced a Bug
- Finds the commit that introduced a bug by performing a binary search through the commit history.
- git bisect start
- git bisect good <last-known-good-commit>
- git bisect bad <bad-commit>
- git clean – Remove Untracked Files
- Over time, your project may accumulate untracked files or directories. To remove them, use:
- git clean -f
- You can also remove untracked directories by adding the -d flag:
- git clean -fd
- git filter-branch – Rewrite Commit History
- Let’s say sensitive information was mistakenly committed to the repository. You can use git filter-branch to remove or modify that information from the entire commit history:
- git filter-branch –tree-filter ‘rm -f sensitive-file.txt’ HEAD
- git blame – Track Changes by Author
- To find out who last modified a specific line in a file, use:
- git blame filename
- git worktree – Manage Multiple Working Directories
- If you need to work on multiple branches simultaneously, you can use git worktree to create an additional working directory:
- git worktree add ../branch-dir feature-branch
- git submodule – Manage Nested Repositories
- Use submodules to keep a Git repository as a subdirectory of another repository. It’s useful when your project relies on other repositories.
- git submodule add <repository-url>
Best Practices for Using Git
- Commit Early, Commit Often
- Why? Committing often keeps your work segmented and helps track changes step by step. For example, if you are working on a large feature, break it down into smaller chunks and commit each part with meaningful messages.
- Use Descriptive Commit Messages
- Why? A clear and concise commit message helps others (and future you) understand the purpose of each commit. For example:
- git commit -m “Changes: Updated tests as per new feature changes”
- Branch Per Feature
- Why? Always work on a new branch for each feature or bug fix. This keeps your main branch stable and makes it easier to test and review the changes in isolation. For example, creating a feature branch:
- git branch feature/user-auth
- Regularly Pull Changes
- Why? Regularly pulling changes from the remote repository helps avoid merge conflicts. If working in a team, it’s essential to stay up-to-date with the latest code.
- Rebase Instead of Merging for Clean History
- Why? Rebasing keeps your project history clean by avoiding unnecessary merge commits. For example, to rebase your feature branch onto main:
- git checkout feature/user-auth
- git rebase main
- Keep the main Branch Clean
- Always aim to keep the main branch deployable and stable. It should only contain thoroughly tested and reviewed code. This avoids the risk of introducing bugs into production environments.
- Use .gitignore to Avoid Unnecessary Files
- Your project may contain files or directories that don’t need to be tracked, such as temporary build files or local configuration settings. The .gitignore file tells Git which files to ignore. For example:
- /node_modules
- .DS_Store
- Squash Commits for a Clean History
- If you’ve made several small commits while working on a feature, consider squashing them before merging the feature branch into main. This keeps your commit history clean and readable. Use:
- git rebase -i HEAD~<number of commits>
- Review Changes Before Committing
- Use git diff to review your changes before committing. This prevents committing unintended modifications:
- git diff
Conclusion
Git is an incredibly powerful tool when used correctly. By mastering both basic and advanced commands, you can efficiently manage your codebase, collaborate with others, and avoid common pitfalls. Adopting best practices such as committing often, using descriptive commit messages, and keeping branches organized will greatly enhance your Git workflow.
As you become more familiar with advanced Git commands, such as git rebase, git cherry-pick, and git bisect, you’ll be equipped to handle even the most complex version control challenges. With these real-world examples, you now have a strong foundation to take your Git expertise to the next level.
Witness how our meticulous approach and cutting-edge solutions elevated quality and performance to new heights. Begin your journey into the world of software testing excellence. To know more refer to Tools & Technologies & QA Services.
If you would like to learn more about the awesome services we provide, be sure to reach out.
Happy testing! 🙂